用c语言写一段程序。该程序是员工管理系统,员工管理系统包含查询物品信息,员工信息,游览信息三部分。游览信息包括通过商品菜单来判断商品是否在打折和查询物品的销售情况。查询物品信息又要包含查询商品编号是否存在,不存在的话要添加编号
时间: 2023-12-28 13:03:55 浏览: 25
以下是一个简单的员工管理系统的C语言代码,包含查询物品信息,员工信息和游览信息三部分。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义员工结构体
typedef struct {
char name[20];
int age;
float salary;
} Employee;
// 定义商品结构体
typedef struct {
int id;
char name[20];
float price;
int on_sale;
int sold;
} Item;
// 定义商品菜单
Item items[] = {
{101, "Apple", 5.0, 1, 50},
{102, "Banana", 2.5, 0, 30},
{103, "Orange", 4.0, 1, 20},
{104, "Grape", 6.5, 0, 10},
};
// 商品数量
int num_items = sizeof(items) / sizeof(Item);
// 查询商品是否在打折
void check_sale(int item_id) {
int i;
for (i = 0; i < num_items; i++) {
if (items[i].id == item_id) {
if (items[i].on_sale) {
printf("商品%s正在打折,当前价格为%.2f元。\n", items[i].name, items[i].price * 0.8);
} else {
printf("商品%s不在打折,当前价格为%.2f元。\n", items[i].name, items[i].price);
}
return;
}
}
printf("商品编号%d不存在。\n", item_id);
}
// 查询物品的销售情况
void check_sold(int item_id) {
int i;
for (i = 0; i < num_items; i++) {
if (items[i].id == item_id) {
printf("商品%s已售出%d件。\n", items[i].name, items[i].sold);
return;
}
}
printf("商品编号%d不存在。\n", item_id);
}
// 查询商品编号是否存在
int check_item(int item_id) {
int i;
for (i = 0; i < num_items; i++) {
if (items[i].id == item_id) {
return i;
}
}
return -1;
}
// 添加商品编号
void add_item(int item_id) {
items[num_items].id = item_id;
printf("请输入商品名称:");
scanf("%s", items[num_items].name);
printf("请输入商品价格:");
scanf("%f", &items[num_items].price);
printf("请输入商品是否在打折(1表示打折,0表示不打折):");
scanf("%d", &items[num_items].on_sale);
items[num_items].sold = 0;
num_items++;
}
// 查询员工信息
void check_employee(Employee *employees, int num_employees) {
int i;
printf("员工信息如下:\n");
printf("姓名\t年龄\t工资\n");
for (i = 0; i < num_employees; i++) {
printf("%s\t%d\t%.2f\n", employees[i].name, employees[i].age, employees[i].salary);
}
}
int main() {
int choice;
int item_id;
int index;
Employee employees[10] = {
{"张三", 25, 5000},
{"李四", 30, 6000},
{"王五", 35, 7000},
{"赵六", 40, 8000},
};
int num_employees = sizeof(employees) / sizeof(Employee);
while (1) {
printf("请选择操作:\n");
printf("1. 查询物品信息\n");
printf("2. 查询员工信息\n");
printf("3. 游览信息\n");
printf("4. 退出程序\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入商品编号:");
scanf("%d", &item_id);
index = check_item(item_id);
if (index == -1) {
printf("该商品编号不存在,是否添加(1表示添加,0表示不添加):");
scanf("%d", &choice);
if (choice) {
add_item(item_id);
}
} else {
check_sale(item_id);
check_sold(item_id);
}
break;
case 2:
check_employee(employees, num_employees);
break;
case 3:
printf("商品菜单如下:\n");
printf("编号\t名称\t价格\t打折\t已售出\n");
for (int i = 0; i < num_items; i++) {
printf("%d\t%s\t%.2f\t%s\t%d\n", items[i].id, items[i].name, items[i].price, items[i].on_sale ? "是" : "否", items[i].sold);
}
break;
case 4:
exit(0);
default:
printf("无效操作,请重新输入。\n");
}
}
return 0;
}
```
上述代码实现了员工管理系统中的查询物品信息,员工信息和游览信息三部分。其中,游览信息包括通过商品菜单来判断商品是否在打折和查询物品的销售情况;查询物品信息又要包含查询商品编号是否存在,不存在的话要添加编号。
相关推荐
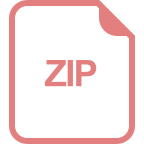
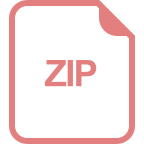
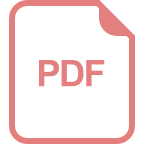
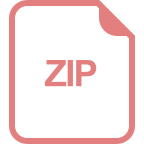
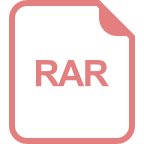
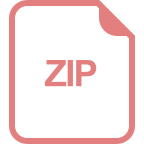
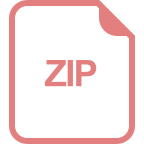
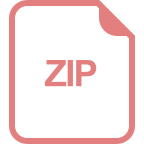