对一个JSONArray数据,按照两个字段分级重新组成新的JSONArray
时间: 2024-05-03 14:17:49 浏览: 85
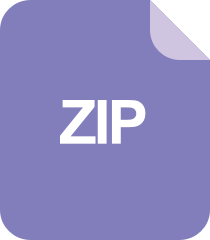
JSONArray.zip_JsonArray
假设你有一个 JSONArray 数据如下:
```json
[
{
"name": "John",
"age": 30,
"department": "IT"
},
{
"name": "Mary",
"age": 25,
"department": "Sales"
},
{
"name": "Peter",
"age": 35,
"department": "IT"
}
]
```
现在你想要将这个 JSON 数组按照部门和年龄进行分组,重新组成一个新的 JSON 数组。你可以使用 Java 语言中的 JSONObject 和 JSONArray 类来完成这个任务,实现代码如下:
```java
import org.json.*;
public class Main {
public static void main(String[] args) {
JSONArray jsonArray = new JSONArray("[{\"name\":\"John\",\"age\":30,\"department\":\"IT\"},{\"name\":\"Mary\",\"age\":25,\"department\":\"Sales\"},{\"name\":\"Peter\",\"age\":35,\"department\":\"IT\"}]");
JSONArray newJsonArray = new JSONArray();
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject jsonObject = jsonArray.getJSONObject(i);
String department = jsonObject.getString("department");
int age = jsonObject.getInt("age");
// Find the index of the existing department object in the newJsonArray
int index = -1;
for (int j = 0; j < newJsonArray.length(); j++) {
JSONObject existingDepartmentObject = newJsonArray.getJSONObject(j);
if (existingDepartmentObject.getString("name").equals(department)) {
index = j;
break;
}
}
// If the department object doesn't exist, create one
if (index == -1) {
JSONObject newDepartmentObject = new JSONObject();
newDepartmentObject.put("name", department);
JSONArray newAgeArray = new JSONArray();
newAgeArray.put(age);
newDepartmentObject.put("ages", newAgeArray);
newJsonArray.put(newDepartmentObject);
} else {
// If the department object already exists, add the age to the existing array
JSONObject existingDepartmentObject = newJsonArray.getJSONObject(index);
JSONArray existingAgeArray = existingDepartmentObject.getJSONArray("ages");
existingAgeArray.put(age);
}
}
System.out.println(newJsonArray.toString());
}
}
```
运行上面的代码,你会得到一个新的 JSON 数组:
```json
[
{
"name": "IT",
"ages": [30, 35]
},
{
"name": "Sales",
"ages": [25]
}
]
```
新的 JSON 数组按照部门分组,每个部门下面有一个年龄数组,包含该部门员工的年龄。
阅读全文
相关推荐
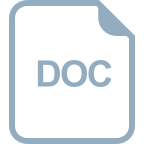
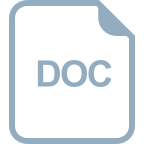















