python subprocess 实现svn文件检出
时间: 2024-06-09 17:06:03 浏览: 182
可以使用Python的subprocess模块来调用svn命令行工具实现文件检出。具体实现步骤如下:
1. 导入subprocess模块
```python
import subprocess
```
2. 构造svn命令行参数
```python
svn_args = ['svn', 'checkout', 'svn://svn.example.com/path/to/repo', '/path/to/local/dir']
```
其中,svn://svn.example.com/path/to/repo是svn仓库的地址,/path/to/local/dir是本地目录路径。
3. 调用subprocess模块执行svn命令
```python
p = subprocess.Popen(svn_args, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
out, err = p.communicate()
```
其中,stdout=subprocess.PIPE和stderr=subprocess.PIPE表示将命令执行过程中的标准输出和标准错误输出都保存到变量中。
4. 输出svn命令执行结果
```python
if err:
print('svn checkout error:', err.decode('utf-8'))
else:
print('svn checkout success')
```
完整代码示例:
```python
import subprocess
svn_args = ['svn', 'checkout', 'svn://svn.example.com/path/to/repo', '/path/to/local/dir']
p = subprocess.Popen(svn_args, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
out, err = p.communicate()
if err:
print('svn checkout error:', err.decode('utf-8'))
else:
print('svn checkout success')
```
阅读全文
相关推荐


















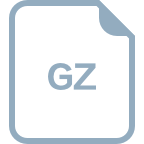