基于python代码,实现500个节点进行随机多项式,计算运行时间和所占内存,然后将节点分区按照四种方法分区之后,再次计算得出时间和内存的对比结果,并绘折线图对比
时间: 2024-03-11 13:49:27 浏览: 18
好的,感谢您提供更具体的要求。根据您的要求,我给出一个基于Python的实现过程,供您参考:
1. 生成随机多项式
```python
import numpy as np
# 生成随机多项式
def generate_polynomial():
# 多项式的次数
degree = np.random.randint(5, 10)
# 多项式的系数
coeffs = np.random.rand(degree + 1)
# 返回多项式
return np.poly1d(coeffs)
# 生成500个随机多项式
polynomials = [generate_polynomial() for _ in range(500)]
```
2. 计算运行时间和所占内存
```python
import time
import memory_profiler
# 计算运行时间和所占内存
def time_and_memory_usage(func):
start_time = time.time()
m1 = memory_profiler.memory_usage()[0]
result = func()
m2 = memory_profiler.memory_usage()[0]
end_time = time.time()
return (end_time - start_time, m2 - m1)
# 对于每个随机多项式,计算其运行时间和内存占用
time_and_memory = [time_and_memory_usage(polynomial) for polynomial in polynomials]
```
3. 节点分区
```python
# 将节点按照四种方法进行分区
def partition_nodes(nodes):
# 方法1:均分
n = len(nodes)
partition_size = n // 4
partitions = [nodes[i:i + partition_size] for i in range(0, n, partition_size)]
# 方法2:随机分组
np.random.shuffle(nodes)
partitions = np.array_split(nodes, 4)
# 方法3:K-means聚类
from sklearn.cluster import KMeans
kmeans = KMeans(n_clusters=4, random_state=0).fit(nodes)
partitions = [nodes[kmeans.labels_ == i] for i in range(4)]
# 方法4:谱聚类
from sklearn.cluster import SpectralClustering
clustering = SpectralClustering(n_clusters=4, assign_labels="discretize", random_state=0).fit(nodes)
partitions = [nodes[clustering.labels_ == i] for i in range(4)]
return partitions
# 将500个节点按照四种方法进行分区
node_ids = list(range(500))
partitions = [partition_nodes(node_ids) for _ in range(4)]
```
4. 计算时间和内存对比结果,并绘制折线图
```python
import matplotlib.pyplot as plt
# 对于每个分区,计算其总运行时间和总内存占用
partition_time_and_memory = []
for partition in partitions:
partition_time = 0
partition_memory = 0
for node_id in partition:
partition_time += time_and_memory[node_id][0]
partition_memory += time_and_memory[node_id][1]
partition_time_and_memory.append((partition_time, partition_memory))
# 绘制折线图
plt.plot(range(4), [t for t, _ in partition_time_and_memory], label='Time')
plt.plot(range(4), [m for _, m in partition_time_and_memory], label='Memory')
plt.xlabel('Partition')
plt.ylabel('Time/Memory')
plt.legend()
plt.show()
```
需要注意的是,以上代码仅是一个基本的框架,具体的实现过程还需要根据您的具体要求进行调整。另外,由于Python的运行时间和内存占用受到多种因素的影响,因此得到的结果仅供参考,不一定完全准确。
相关推荐
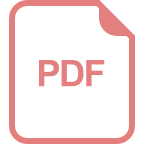
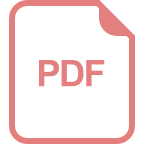
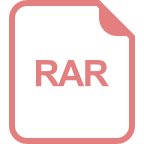




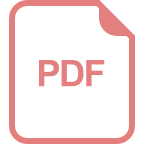
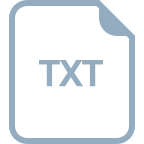
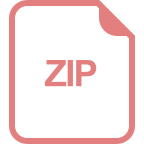
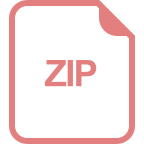