LSA/LSI/LDA算法,关键词提取,python代码
时间: 2023-03-14 20:59:58 浏览: 162
LSA (Latent Semantic Analysis)、LSI (Latent Semantic Indexing) 和 LDA (Latent Dirichlet Allocation) 都是用于文本挖掘和信息检索的算法。它们的目的是从文本中提取关键词,并对文本进行主题建模。
LSA 和 LSI 都是基于矩阵分解的方法,用于提取文本的主题信息。它们的工作原理类似,都是通过对文本中的单词进行统计,并将单词出现的频率转化为权重,然后构建文档-单词矩阵,并对该矩阵进行奇异值分解。通过对分解后的矩阵进行重构,可以得到新的文档-主题矩阵和主题-单词矩阵。新的文档-主题矩阵中的每一行表示一篇文档,每一列表示一个主题,每个元素表示该文档和该主题的相关性。新的主题-单词矩阵中的每一行表示一个主题,每一列表示一个单词,每个元素表示该主题和该单词的相关性。
LDA 是一种生成模型,它通过假设文本由若干个主题构成,并假设每个主题都有一个单词分布,来提取文本的主题信息。LDA 算法的工作原理是,首先
相关问题
LSA/LSI/LDA算法,关键词提取,python代码,直接写代码不解释
LSA (Latent Semantic Analysis),LSI (Latent Semantic Indexing) 和 LDA (Latent Dirichlet Allocation) 都是用于文本处理的有监督学习算法。它们的目的是通过对文本进行降维处理,从而提取出文本中的关键词。
这些算法的具体实现方法略有不同,但它们的基本原理都是通过使用矩阵分解和奇异值分解来提取文本的主要特征。
下面是使用 Python 实现 LSA 算法的代码示例:
```python
from sklearn.decomposition import TruncatedSVD
from sklearn.feature_extraction.text import TfidfVectorizer
def extract_keywords(documents):
# 对文本进行 tf-idf 特征提取
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(documents)
# 使用 LSA 算法进行降维
svd = TruncatedSVD(n_components=100)
X_reduced = svd.fit_transform(X)
# 获取每个文档的关键词
keywords = []
for i, document in enumerate(documents):
row = X_reduced[i, :]
terms = vectorizer.inverse_transform(row)
keywords.append(terms[0])
return keywords
# 示例:提取一组文档的关键词
documents = [
"This is the first document.",
"This document is the second document.",
"And this is the third one.",
"Is this the first document?",
]
keywords = extract_keywords(documents)
print(keywords)
```
上述代码使用了 scikit-learn 中的 TruncatedSVD 和 TfidfVectorizer 类来实现 LSA 算法。TfidfVectorizer 类用于将文本转换为 tf-idf 特征矩阵,TruncatedSVD 类用于对 tf-idf 特征矩阵进行奇异值分解并
Python库中还有哪些可以实现TF-IDF、TextRank和LSA三种算法的程序包,并通过实例实现关键词提取。
关于TF-IDF、TextRank和LSA三种算法实现关键词提取的Python程序包,常用的包有:
1. sklearn,它提供了TfidfVectorizer和CountVectorizer等工具,可以实现TF-IDF算法。同时,它也提供了LSA和LDA等主题模型算法。
2. nltk,它是Python自然语言处理的一个常用工具库,其中包含了TextRank算法的实现。
3. gensim,它是一个用于处理文本的Python工具库,提供了LSI(Latent Semantic Indexing,潜在语义分析)和LDA等算法实现。
4. jieba,它是一个中文分词工具库,支持TF-IDF算法。
这些工具库都提供了详细的文档和示例,可以很方便地实现关键词提取。例如,使用sklearn的TfidfVectorizer实现TF-IDF算法的关键词提取代码如下:
```
from sklearn.feature_extraction.text import TfidfVectorizer
texts = ['This is a text', 'Another text', 'Yet another text']
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(texts)
feature_names = vectorizer.get_feature_names()
doc = 0
feature_index = tfidf_matrix[doc,:].nonzero()[1]
tfidf_scores = zip(feature_index, [tfidf_matrix[doc,x] for x in feature_index])
top_keywords = sorted(tfidf_scores, key=lambda x: x[1], reverse=True)[:5]
print(top_keywords)
```
这段代码中,首先使用TfidfVectorizer将文本矩阵转换为TF-IDF矩阵,然后通过get_feature_names方法获取特征名列表,使用nonzero方法获取第0个文本的非零元素下标,通过zip将特征下标和对应的TF-IDF分数打包为元组。最后,使用sorted方法将元组按分数从大到小排序,并选择前5个元组,输出作为关键词。
类似地,使用gensim库的LSI算法实现关键词提取的代码如下:
```
from gensim import corpora
from gensim.models import LsiModel
texts = [['This', 'is', 'a', 'text'], ['Another', 'text'], ['Yet', 'another', 'text']]
dictionary = corpora.Dictionary(texts)
corpus = [dictionary.doc2bow(text) for text in texts]
lsi_model = LsiModel(corpus, num_topics=2)
lsi_matrix = lsi_model[corpus]
doc = 0
top_keywords = sorted(lsi_matrix[doc], key=lambda x: -x[1])[:5]
print(top_keywords)
```
这段代码中,首先使用corpora.Dictionary将文本列表转换为词典,再使用doc2bow方法将每个文本转换为词袋向量表示。然后,使用LsiModel训练得到一个2维的LSI模型,对文本矩阵进行转换得到LSI矩阵。最后,使用sorted方法将LSI矩阵中第0个文本的元素按LSI分数从大到小排序,并选择前5个元素,输出作为关键词。
使用这些工具库,可以很方便地实现关键词提取并进行文本分析。
阅读全文
相关推荐
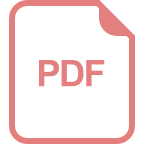
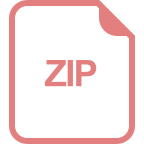
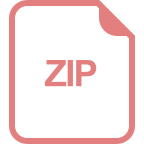
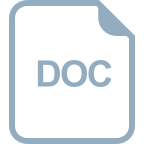
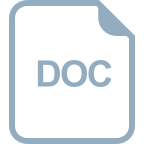
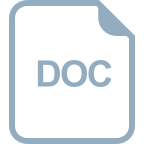
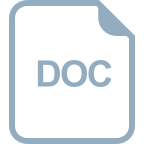
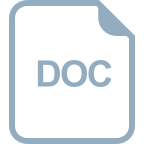
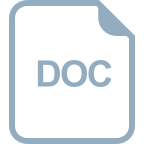
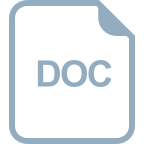
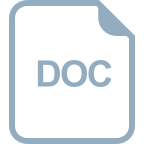
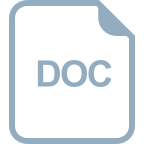
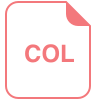

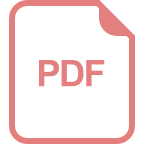