写一份html代码,实现定义一个全局数组变量,并在网页中输出打印这些元素
时间: 2024-04-10 10:28:49 浏览: 9
<!DOCTYPE html>
<html>
<head>
<title>Global Array Variable</title>
</head>
<body>
<script>
// 定义全局数组变量
var globalArray = [1, 2, 3, 4, 5];
// 输出打印数组元素
document.write("数组元素为: " + globalArray);
</script>
</body>
</html>
相关问题
写一份html代码,实现定义一个全局数组变量,并在body中使用h1标签一个一个的中输出这些元素
<!DOCTYPE html>
<html>
<head>
<title>Global Array Variable</title>
</head>
<body>
<h1 id="output"></h1>
<script>
// 定义全局数组变量
var globalArray = [1, 2, 3, 4, 5];
// 使用循环输出数组元素
for (var i = 0; i < globalArray.length; i++) {
document.getElementById("output").innerHTML += globalArray[i] + "<br>";
}
</script>
</body>
</html>
代码生成 利用C语言写一个户籍管理系统,
好的,我可以给你提供一份简单的C语言代码示例,实现一个基本的户籍管理系统。这个系统包括以下几个功能:添加人员信息、修改人员信息、查询人员信息和删除人员信息。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAXSIZE 100 // 最大人员数量
// 定义一个结构体,表示一个人员的信息
struct Person {
char name[20]; // 姓名
char sex[5]; // 性别
int age; // 年龄
char id[20]; // 身份证号
};
// 定义一个全局变量,表示当前系统中的人员数量
int person_num = 0;
// 定义一个数组,用来存储所有人员的信息
struct Person person_list[MAXSIZE];
// 添加人员信息
void add_person() {
if (person_num >= MAXSIZE) {
printf("人员数量已达到上限,无法添加新的人员信息!\n");
return;
}
struct Person p;
printf("请输入人员姓名:");
scanf("%s", p.name);
printf("请输入人员性别:");
scanf("%s", p.sex);
printf("请输入人员年龄:");
scanf("%d", &p.age);
printf("请输入人员身份证号:");
scanf("%s", p.id);
person_list[person_num] = p;
person_num++;
printf("人员信息添加成功!\n");
}
// 修改人员信息
void modify_person() {
char id[20];
printf("请输入要修改的人员身份证号:");
scanf("%s", id);
int index = -1;
for (int i = 0; i < person_num; i++) {
if (strcmp(person_list[i].id, id) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("未找到指定的人员信息!\n");
return;
}
struct Person p;
printf("请输入修改后的人员姓名:");
scanf("%s", p.name);
printf("请输入修改后的人员性别:");
scanf("%s", p.sex);
printf("请输入修改后的人员年龄:");
scanf("%d", &p.age);
printf("请输入修改后的人员身份证号:");
scanf("%s", p.id);
person_list[index] = p;
printf("人员信息修改成功!\n");
}
// 查询人员信息
void query_person() {
char id[20];
printf("请输入要查询的人员身份证号:");
scanf("%s", id);
int index = -1;
for (int i = 0; i < person_num; i++) {
if (strcmp(person_list[i].id, id) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("未找到指定的人员信息!\n");
return;
}
printf("姓名:%s\n", person_list[index].name);
printf("性别:%s\n", person_list[index].sex);
printf("年龄:%d\n", person_list[index].age);
printf("身份证号:%s\n", person_list[index].id);
}
// 删除人员信息
void delete_person() {
char id[20];
printf("请输入要删除的人员身份证号:");
scanf("%s", id);
int index = -1;
for (int i = 0; i < person_num; i++) {
if (strcmp(person_list[i].id, id) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("未找到指定的人员信息!\n");
return;
}
for (int i = index; i < person_num - 1; i++) {
person_list[i] = person_list[i + 1];
}
person_num--;
printf("人员信息删除成功!\n");
}
int main() {
while (1) {
int choice;
printf("1. 添加人员信息\n");
printf("2. 修改人员信息\n");
printf("3. 查询人员信息\n");
printf("4. 删除人员信息\n");
printf("5. 退出系统\n");
printf("请选择功能:");
scanf("%d", &choice);
switch (choice) {
case 1:
add_person();
break;
case 2:
modify_person();
break;
case 3:
query_person();
break;
case 4:
delete_person();
break;
case 5:
printf("感谢使用本系统,再见!\n");
return 0;
default:
printf("无效的选择,请重新选择!\n");
break;
}
}
}
```
以上就是一个简单的户籍管理系统的C语言代码示例,可以根据需要进行修改和完善。
相关推荐
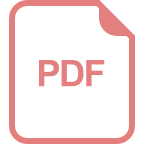
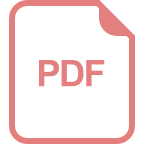
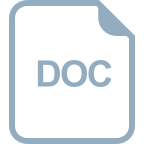












