python与tkinter实现鼠标点击与记录鼠标和回放
时间: 2024-05-14 17:18:13 浏览: 56
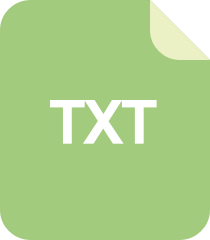
python与tkinter编程

可以使用Python的Tkinter模块来实现鼠标点击和记录鼠标操作的功能,具体步骤如下:
1. 导入Tkinter模块和time模块。
```python
import tkinter as tk
import time
```
2. 创建一个Tkinter窗口,并设置窗口标题和大小。
```python
win = tk.Tk()
win.title("Mouse Recorder and Playback")
win.geometry("400x300")
```
3. 创建一个Canvas对象,并添加到窗口中。
```python
canvas = tk.Canvas(win, bg="white")
canvas.pack(expand=True, fill="both")
```
4. 定义鼠标点击事件的回调函数,并在Canvas上绑定该事件。
```python
def on_click(event):
x, y = event.x, event.y
canvas.create_oval(x-5, y-5, x+5, y+5, fill="red")
actions.append((time.time(), "click", x, y))
canvas.bind("<Button-1>", on_click)
```
5. 定义记录鼠标操作的函数。
```python
def record():
global recording, actions
recording = True
actions = []
canvas.delete("all")
while recording:
win.update()
with open("actions.txt", "w") as f:
for a in actions:
f.write("{},{},{},{}\n".format(a[0], a[1], a[2], a[3]))
```
6. 定义回放鼠标操作的函数。
```python
def playback():
canvas.delete("all")
with open("actions.txt", "r") as f:
for line in f:
ts, action, x, y = line.strip().split(",")
x, y = int(x), int(y)
delay = float(ts) - actions[0][0]
time.sleep(delay)
if action == "click":
canvas.create_oval(x-5, y-5, x+5, y+5, fill="blue")
canvas.update()
```
7. 在窗口中添加两个按钮,分别用于开始记录和回放鼠标操作。
```python
record_btn = tk.Button(win, text="Record", command=record)
record_btn.pack(side="left")
playback_btn = tk.Button(win, text="Playback", command=playback)
playback_btn.pack(side="right")
```
完整代码如下:
```python
import tkinter as tk
import time
win = tk.Tk()
win.title("Mouse Recorder and Playback")
win.geometry("400x300")
canvas = tk.Canvas(win, bg="white")
canvas.pack(expand=True, fill="both")
def on_click(event):
x, y = event.x, event.y
canvas.create_oval(x-5, y-5, x+5, y+5, fill="red")
actions.append((time.time(), "click", x, y))
canvas.bind("<Button-1>", on_click)
def record():
global recording, actions
recording = True
actions = []
canvas.delete("all")
while recording:
win.update()
with open("actions.txt", "w") as f:
for a in actions:
f.write("{},{},{},{}\n".format(a[0], a[1], a[2], a[3]))
def playback():
canvas.delete("all")
with open("actions.txt", "r") as f:
for line in f:
ts, action, x, y = line.strip().split(",")
x, y = int(x), int(y)
delay = float(ts) - actions[0][0]
time.sleep(delay)
if action == "click":
canvas.create_oval(x-5, y-5, x+5, y+5, fill="blue")
canvas.update()
record_btn = tk.Button(win, text="Record", command=record)
record_btn.pack(side="left")
playback_btn = tk.Button(win, text="Playback", command=playback)
playback_btn.pack(side="right")
win.mainloop()
```
阅读全文
相关推荐
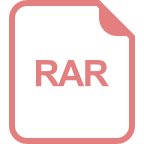
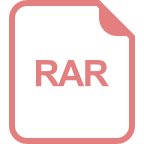
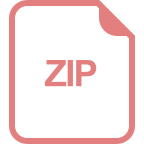
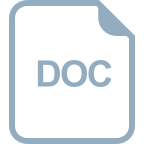
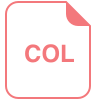


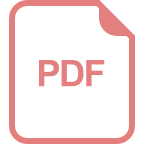
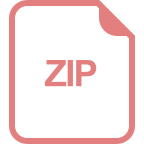
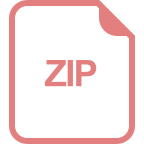
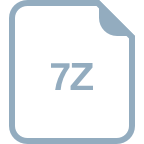
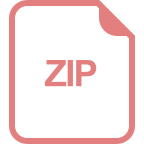