java用exchange online发送邮件
时间: 2023-09-05 14:11:44 浏览: 167
在Java中使用Exchange Online发送邮件,你可以使用JavaMail API来完成。下面是一个简单的示例代码:
```java
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class ExchangeOnlineEmailSender {
public static void main(String[] args) {
// 配置SMTP服务器和认证信息
Properties props = new Properties();
props.put("mail.smtp.host", "smtp.office365.com");
props.put("mail.smtp.port", "587");
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
// 设置发件人邮箱和密码
final String username = "your_email@your_domain.com";
final String password = "your_password";
// 创建会话
Session session = Session.getInstance(props, new javax.mail.Authenticator() {
protected javax.mail.PasswordAuthentication getPasswordAuthentication() {
return new javax.mail.PasswordAuthentication(username, password);
}
});
try {
// 创建邮件消息
Message message = new MimeMessage(session);
// 设置发件人
message.setFrom(new InternetAddress("your_email@your_domain.com"));
// 设置收件人
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse("recipient_email@recipient_domain.com"));
// 设置邮件主题
message.setSubject("Hello from JavaMail");
// 设置邮件正文
message.setText("This is a test email from JavaMail API");
// 发送邮件
Transport.send(message);
System.out.println("Email sent successfully!");
} catch (MessagingException e) {
e.printStackTrace();
}
}
}
```
请注意,你需要将代码中的`your_email@your_domain.com`替换为你的发件人邮箱地址,`your_password`替换为你的邮箱密码,`recipient_email@recipient_domain.com`替换为接收邮件的收件人邮箱地址。
此代码使用SMTP协议通过Exchange Online发送邮件。它会连接到Office 365的SMTP服务器,并使用提供的用户名和密码进行身份验证。确保你已经启用了Exchange Online中的SMTP并拥有有效的SMTP凭据。
此外,确保你已经包含了JavaMail API库,可以从JavaMail官方网站下载并添加到你的项目中。
希望对你有所帮助!
阅读全文
相关推荐
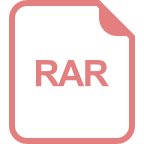
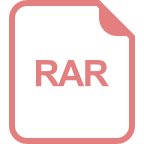
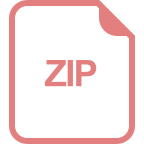
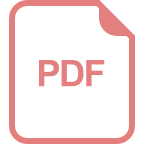
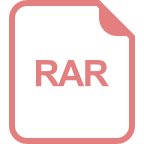
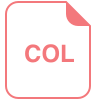
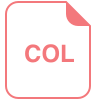
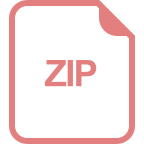
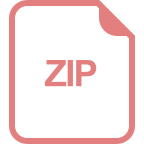