Android studio如何读取checkbox的值
时间: 2024-05-09 14:21:36 浏览: 6
Android Studio 可以通过以下代码读取 checkbox 的值:
```
CheckBox checkBox = findViewById(R.id.checkbox_id);
boolean isChecked = checkBox.isChecked();
```
其中,`checkbox_id` 是你在布局文件中指定的 checkbox 的 id。如果 `isChecked` 为 `true`,则表示 checkbox 被选中了。
相关问题
Android studio如何读取checkbox是否选中
您可以使用以下代码来读取 Android Studio 中的 checkbox 是否被选中:
CheckBox checkBox = findViewById(R.id.checkbox_id);
boolean isChecked = checkBox.isChecked();
其中,checkbox_id 是您在布局文件中为 checkbox 指定的 ID。isChecked() 方法将返回一个布尔值,指示 checkbox 是否被选中。
android studio 登录界面记住密码
在Android Studio中实现登录界面记住密码的功能,可以通过使用SharedPreferences来保存用户的登录信息。
首先,在登录界面的布局文件中,添加一个复选框用于用户选择是否记住密码。如:
```xml
<CheckBox
android:id="@+id/rememberPasswordCheckBox"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="记住密码" />
```
然后,在登录按钮的点击事件中,判断记住密码复选框的状态。如果选择了记住密码,则将用户名和密码保存到SharedPreferences中。代码如下:
```java
Button loginButton = findViewById(R.id.loginButton);
loginButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText usernameEditText = findViewById(R.id.usernameEditText);
EditText passwordEditText = findViewById(R.id.passwordEditText);
CheckBox rememberPasswordCheckBox = findViewById(R.id.rememberPasswordCheckBox);
String username = usernameEditText.getText().toString();
String password = passwordEditText.getText().toString();
if (rememberPasswordCheckBox.isChecked()) {
SharedPreferences preferences = getSharedPreferences("loginPrefs", MODE_PRIVATE);
SharedPreferences.Editor editor = preferences.edit();
editor.putString("username", username);
editor.putString("password", password);
editor.apply();
}
// 登录操作...
}
});
```
在登录界面的`onCreate()`方法中,通过读取SharedPreferences中保存的用户名和密码,并设置到对应的EditText中。代码如下:
```java
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
EditText usernameEditText = findViewById(R.id.usernameEditText);
EditText passwordEditText = findViewById(R.id.passwordEditText);
CheckBox rememberPasswordCheckBox = findViewById(R.id.rememberPasswordCheckBox);
SharedPreferences preferences = getSharedPreferences("loginPrefs", MODE_PRIVATE);
String savedUsername = preferences.getString("username", "");
String savedPassword = preferences.getString("password", "");
usernameEditText.setText(savedUsername);
passwordEditText.setText(savedPassword);
rememberPasswordCheckBox.setChecked(!TextUtils.isEmpty(savedUsername) && !TextUtils.isEmpty(savedPassword));
}
```
通过上述步骤,就可以实现在Android Studio中登录界面记住密码的功能。当用户选择记住密码后,下次打开应用时会直接显示之前保存的用户名和密码,并且可以直接进行登录操作。
相关推荐
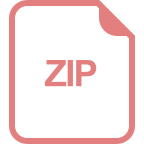









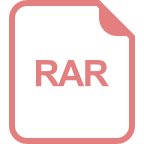