java中统计英文单词数_统计单词数 Java
时间: 2023-10-23 08:25:30 浏览: 1155
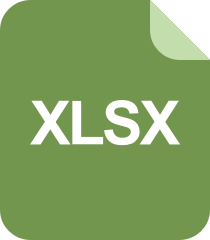
java常用单词统计

好的,我可以为您提供一些关于Java中统计英文单词数的方法。
方法一:使用Split()函数和正则表达式
可以通过使用Split()函数和正则表达式来将字符串按照空格分割成单词,然后统计单词数量。例如:
```java
public static int countWords(String text) {
String[] words = text.split("\\s+");
return words.length;
}
```
这个方法中,使用了正则表达式"\\s+",表示匹配任意空白字符(空格、制表符、换行符等),进行分割。然后返回单词数组的长度即可。
方法二:使用Java8中的Stream API
在Java8中,可以使用Stream API来进行单词的统计。例如:
```java
public static long countWords(String text) {
return Arrays.stream(text.split("\\s+"))
.filter(word -> word.matches("[a-zA-Z]+"))
.count();
}
```
这个方法中,首先使用Split()函数和正则表达式将字符串按照空格分割成单词,然后使用Stream API对每个单词进行过滤,只保留由字母组成的单词,最后返回统计结果。
以上两种方法都可以实现统计英文单词数的功能,您可以根据实际需要选择使用。
阅读全文
相关推荐
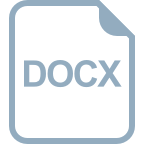
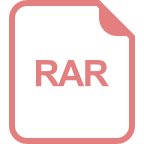

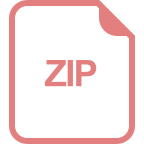
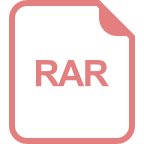



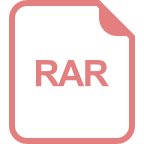
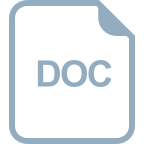





