if (name.indexOf(".") != -1) { updatePower(Integer.parseInt(name.split("\\.")[1])); } // do some random walk else if (power < 100) { updatePower(power + Math.random() * 100); } else if (power > 300) { updatePower(power - Math.random() * 100); } else { updatePower(power + Math.random() * 40 - 20); } how to test this part function, please give me code
时间: 2024-03-22 19:40:14 浏览: 23
To test this function, you can write unit tests using a testing framework such as JUnit. Here is an example of a test class that tests the behavior of the function:
```
import static org.junit.Assert.*;
import org.junit.Test;
public class PowerUpdateTest {
@Test
public void testUpdatePowerWithDot() {
// Test updating power with a name containing a dot
PowerUpdate pu = new PowerUpdate();
pu.setName("test.50");
pu.setPower(200);
pu.updatePower(0);
assertEquals(50, pu.getPower(), 0.01);
}
@Test
public void testUpdatePowerWithLowPower() {
// Test updating power when power is less than 100
PowerUpdate pu = new PowerUpdate();
pu.setName("test");
pu.setPower(50);
pu.updatePower(0);
assertTrue(pu.getPower() >= 50 && pu.getPower() <= 150);
}
@Test
public void testUpdatePowerWithHighPower() {
// Test updating power when power is greater than 300
PowerUpdate pu = new PowerUpdate();
pu.setName("test");
pu.setPower(350);
pu.updatePower(0);
assertTrue(pu.getPower() >= 250 && pu.getPower() <= 350);
}
@Test
public void testUpdatePowerWithMediumPower() {
// Test updating power when power is between 100 and 300
PowerUpdate pu = new PowerUpdate();
pu.setName("test");
pu.setPower(200);
pu.updatePower(0);
assertTrue(pu.getPower() >= 180 && pu.getPower() <= 220);
}
}
```
This test class creates an instance of the `PowerUpdate` class and sets the name and initial power values. It then calls the `updatePower` method with a dummy value and checks the result using `assertEquals` or `assertTrue` depending on the test case. You can run these tests using a testing framework in your IDE or using a build tool such as Maven.
相关推荐
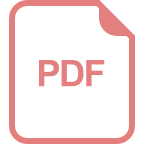
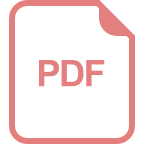
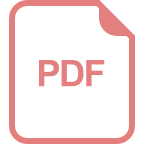









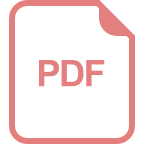
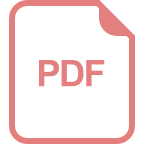
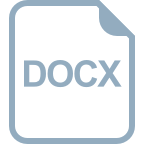
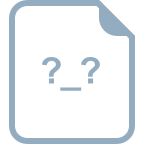
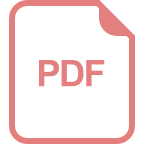
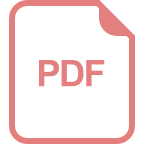