python中configparser模块中ConfigParser().has_option()函数的作用?所需参数?
时间: 2024-05-02 15:23:46 浏览: 12
ConfigParser().has_option()函数的作用是检查指定的section中是否存在指定的option,若存在返回True,否则返回False。
该函数需要两个参数,第一个参数为要检查的section,第二个参数为要检查的option。例如:
```
import configparser
config = configparser.ConfigParser()
config.read('example.ini')
if config.has_option('Section1', 'Option1'):
print('Option1 exists in Section1')
else:
print('Option1 does not exist in Section1')
```
输出结果根据配置文件example.ini而定。
相关问题
python中configparser模块中ConfigParser().has_section()函数的作用?所需参数?
ConfigParser().has_section()函数的作用是检查配置文件中是否存在指定的section。它的参数是section的名称,也就是配置文件中用方括号包括起来的部分的名称。如果存在指定的section,返回True;否则返回False。例如:
```
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
if config.has_section('global'):
print('config.ini 包含 global section')
else:
print('config.ini 不包含 global section')
```
在上面的例子中,我们首先读入了一个配置文件 config.ini,然后使用 has_section() 函数检查是否存在名为 'global' 的 section。如果存在,则输出config.ini 包含 global section;否则,输出config.ini 不包含 global section。
python中configparser模块中ConfigParser().add_section()函数的作用?所需参数?简单案例?
ConfigParser().add_section()函数的作用是添加一个新的配置文件区域。它需要一个参数作为新区域的名称。
一个简单的案例是:
```
import configparser
config = configparser.ConfigParser()
# 添加一个新的区域
config.add_section('Section1')
# 设置区域的值
config.set('Section1', 'key1', 'value1')
config.set('Section1', 'key2', 'value2')
# 保存配置文件
with open('example.ini', 'w') as f:
config.write(f)
```
这段代码创建一个新的配置文件对象,添加了一个名为“Section1”的区域,并设置了两个键值对。最后将配置文件写入到example.ini文件中。
相关推荐
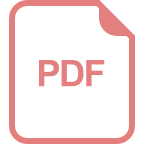
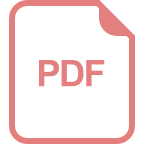












