用C++写出解决下列问题的代码,设计两个基类:教师类(Teacher)、干部类(Cadre),采用多重继承派生出新类: Teacher_Cadre 1、两个基类都包含:姓名、年龄、性别、地址、电话等; 在 Teacher 类中包含:title(职称),在Cadre 类中包含:post(职务),在Teacher_Cadre 中包含:wages(工资); 2、两个基类都包含:display()函数,分别输出本类中的成员信息; 3、在两个基类中的姓名、年龄、性别、地址、电话等数据成员使用相同名字,在派生 类中引用这些数据成员时,采用指定作用域的方式; 4、在派生类Teacher_Cadre 的成员函数Show()中调用Teacher 类中的display()函 数,输出姓名、年龄、性别、地址、电话; 5、使用对象数组保存输入的对象;
时间: 2024-03-31 08:36:00 浏览: 57
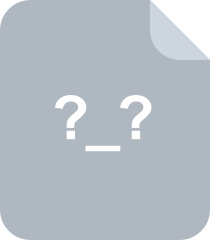
分别声明Teacher(教师)类和Cadre(干部)类,采用多重继承方式由这两个类派生出新类Teacher_Cadre(教师兼干部)。要求:①在两个基类中都包含
下面是代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Teacher {
protected:
string name;
int age;
string gender;
string address;
string phone;
string title;
public:
Teacher(string n, int a, string g, string add, string p, string t):
name(n), age(a), gender(g), address(add), phone(p), title(t) {}
void display() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Gender: " << gender << endl;
cout << "Address: " << address << endl;
cout << "Phone: " << phone << endl;
cout << "Title: " << title << endl;
}
};
class Cadre {
protected:
string name;
int age;
string gender;
string address;
string phone;
string post;
public:
Cadre(string n, int a, string g, string add, string p, string pst):
name(n), age(a), gender(g), address(add), phone(p), post(pst) {}
void display() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Gender: " << gender << endl;
cout << "Address: " << address << endl;
cout << "Phone: " << phone << endl;
cout << "Post: " << post << endl;
}
};
class Teacher_Cadre : public Teacher, public Cadre {
private:
double wages;
public:
Teacher_Cadre(string n, int a, string g, string add, string p, string t, string pst, double w):
Teacher(n, a, g, add, p, t), Cadre(n, a, g, add, p, pst), wages(w) {}
void Show() {
Teacher::display();
}
};
int main() {
const int SIZE = 2;
Teacher_Cadre tc[SIZE] = {
Teacher_Cadre("Tom", 30, "Male", "Beijing", "123456", "Associate Professor", "Chairman", 10000.0),
Teacher_Cadre("Mary", 25, "Female", "Shanghai", "654321", "Assistant Professor", "Secretary", 8000.0)
};
for (int i = 0; i < SIZE; i++) {
tc[i].Show();
cout << endl;
}
return 0;
}
```
这个程序定义了两个基类:`Teacher`和`Cadre`,都包含姓名、年龄、性别、地址和电话等信息,并且都有一个`display()`函数用于输出这些信息。`Teacher`类还包含职称信息,`Cadre`类则包含职务信息。然后通过多重继承,派生出一个新类`Teacher_Cadre`,并在其中增加一个`wages`成员用于记录工资信息。`Teacher_Cadre`类中的`Show()`函数调用`Teacher`类的`display()`函数,以输出姓名、年龄、性别、地址和电话信息。
在`main()`函数中,定义了一个长度为2的`Teacher_Cadre`数组,保存了两个对象的信息,并且输出了每个对象的信息。
阅读全文
相关推荐

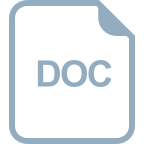
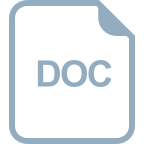





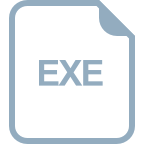
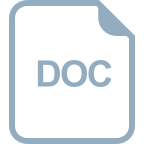
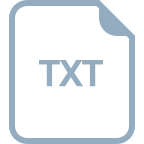
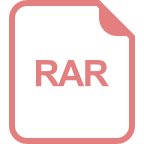
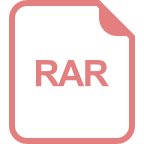
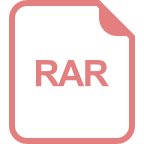
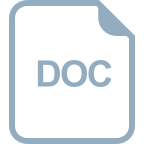
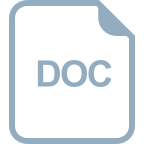
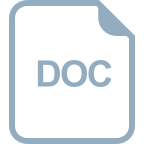