一字符串数组与6个字符串数组比较,如果字符串数组与其它六个字符串中的一个相同则返回true,如果都不同则返回false
时间: 2024-12-19 22:26:20 浏览: 3
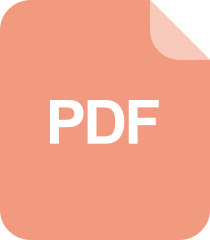
c#实现从字符串数组中把数字的元素找出来
要实现这个功能,你可以遍历那六个字符串数组,并使用标准库中的字符串比较函数,如 C 语言的 strcmp() 或 C++ 的 std::string 的 compare() 方法。下面是一个简单的示例,假设我们有一个名为 `array` 的字符串数组,而另外六个分别是 `arr1`, `arr2`, ..., `arr6`。你可以创建一个函数来进行比较:
```cpp
#include <vector>
#include <string>
bool stringArrayCompare(std::vector<std::string> array, std::string arr1, std::string arr2, std::string arr3, std::string arr4, std::string arr5, std::string arr6) {
for (const auto& str : array) {
bool matchFound = false;
for (int i = 1; i <= 6; i++) { // 遍历6个额外数组
if (str == arr[i]) {
matchFound = true;
break;
}
}
if (matchFound)
return true; // 如果找到匹配,立即返回 true
}
return false; // 遍历完都没找到匹配,返回 false
}
```
或者,如果你是在 C 语言环境下:
```c
#include <stdio.h>
#include <stdbool.h>
#include <stdlib.h>
#include <string.h>
bool compare_strings(char str[], char* arr[], int num_arrays) {
for (int i = 0; i < num_arrays; i++) {
if (strcmp(str, arr[i]) == 0) {
return true;
}
}
return false;
}
bool compare_string_array(char** array, char* arr1[], char* arr2[], char* arr3[], char* arr4[], char* arr5[], char* arr6) {
for (size_t i = 0; array[i] != NULL; i++) {
if (compare_strings(array[i], arr1, 6)) {
return true;
}
}
return false;
}
```
在这个例子中,`compare_strings()` 函数会检查是否找到了匹配的字符串,如果找到就返回真,`compare_string_array()` 则负责遍历整个给定的字符串数组。
阅读全文
相关推荐
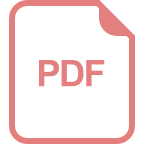
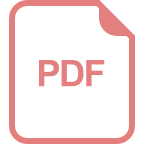


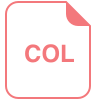
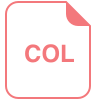
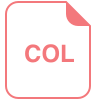
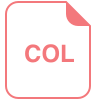
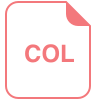
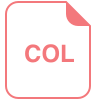







