qt写的tcp客户端程序实现简单的接受发送数据
时间: 2024-01-24 21:03:34 浏览: 27
以下是一个使用 Qt 编写的 TCP 客户端程序,可以实现简单的接收和发送数据:
```cpp
#include <QtWidgets/QApplication>
#include <QtWidgets/QWidget>
#include <QtNetwork/QTcpSocket>
#include <QtWidgets/QHBoxLayout>
#include <QtWidgets/QTextEdit>
#include <QtWidgets/QPushButton>
#include <QtWidgets/QLineEdit>
#include <QtWidgets/QMessageBox>
class TcpClient : public QWidget {
Q_OBJECT
public:
TcpClient() {
// 构造界面
QHBoxLayout *layout = new QHBoxLayout(this);
m_textEdit = new QTextEdit(this);
layout->addWidget(m_textEdit);
QVBoxLayout *vLayout = new QVBoxLayout();
layout->addLayout(vLayout);
m_lineEdit = new QLineEdit(this);
vLayout->addWidget(m_lineEdit);
m_sendButton = new QPushButton("发送", this);
vLayout->addWidget(m_sendButton);
// 连接信号和槽
connect(m_sendButton, &QPushButton::clicked, this, &TcpClient::sendData);
// 创建 TcpSocket
m_tcpSocket = new QTcpSocket(this);
connect(m_tcpSocket, &QTcpSocket::readyRead, this, &TcpClient::readData);
connect(m_tcpSocket, &QTcpSocket::disconnected, this, &TcpClient::disconnectFromServer);
// 连接服务器
m_tcpSocket->connectToHost("127.0.0.1", 8888);
if (!m_tcpSocket->waitForConnected()) {
QMessageBox::critical(this, "错误", "连接服务器失败");
}
}
private slots:
void sendData() {
QString message = m_lineEdit->text();
if (message.isEmpty()) {
return;
}
m_tcpSocket->write(message.toUtf8());
m_tcpSocket->flush();
m_lineEdit->clear();
}
void readData() {
QByteArray data = m_tcpSocket->readAll();
m_textEdit->append(QString(data));
}
void disconnectFromServer() {
QMessageBox::information(this, "提示", "与服务器断开连接");
}
private:
QTcpSocket *m_tcpSocket;
QTextEdit *m_textEdit;
QLineEdit *m_lineEdit;
QPushButton *m_sendButton;
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
TcpClient client;
client.show();
return app.exec();
}
#include "main.moc"
```
在这个程序中,我们使用了 QTcpSocket 类来实现 TCP 客户端。在构造函数中,我们首先创建了界面,并连接了发送按钮的 clicked 信号和 sendData 槽。然后我们创建了 QTcpSocket 对象,并连接了 readyRead 和 disconnected 信号与 readData 和 disconnectFromServer 槽。最后,我们使用 connectToHost 函数连接到服务器。如果连接失败,我们弹出一个错误对话框。
sendData 槽中,我们首先读取了用户在 QLineEdit 中输入的字符串,并检查是否为空。如果不为空,我们使用 write 函数将字符串发送给服务器,然后清空 QLineEdit。
readData 槽中,我们首先使用 readAll 函数读取从服务器传回的数据,并将其转换为 QString,然后使用 append 函数将其添加到 QTextEdit 中。
disconnectFromServer 槽中,我们弹出一个提示对话框,告诉用户与服务器已经断开连接。
在 main 函数中,我们创建了 QApplication 和 TcpClient 对象,并将 TcpClient 对象显示出来。最后,我们调用 app.exec() 进入 Qt 的事件循环。
相关推荐
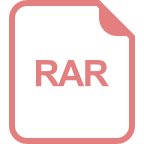
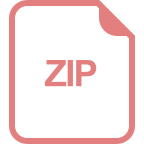
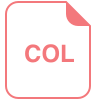
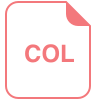












