用html,js,css实现一个评论区
时间: 2024-03-09 08:33:43 浏览: 116
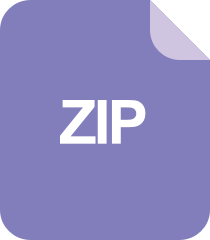
评论功能区
<!DOCTYPE html>
<html>
<head>
<title>评论区</title>
<style type="text/css">
.comment-section {
border: 1px solid #ccc;
padding: 10px;
margin-bottom: 20px;
}
.comment-section h3 {
margin-top: 0;
font-size: 18px;
font-weight: bold;
}
.comment-section p {
margin-top: 0;
font-size: 16px;
line-height: 1.5;
}
.comment-form {
margin-top: 20px;
}
.comment-form label {
display: block;
font-weight: bold;
margin-bottom: 5px;
}
.comment-form input,
.comment-form textarea {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
}
.comment-form button {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
}
.comment-form button:hover {
background-color: #45a049;
}
</style>
</head>
<body>
<div class="comment-section">
<h3>最新评论</h3>
<p>暂无评论</p>
</div>
<div class="comment-form">
<h3>发表评论</h3>
<form>
<label for="name">姓名</label>
<input type="text" id="name" name="name" placeholder="请输入您的姓名" required>
<label for="email">邮箱</label>
<input type="email" id="email" name="email" placeholder="请输入您的邮箱" required>
<label for="comment">评论</label>
<textarea id="comment" name="comment" placeholder="请输入您的评论" required></textarea>
<button type="submit" onclick="addComment()">提交评论</button>
</form>
</div>
<script type="text/javascript">
// 添加评论函数
function addComment() {
// 获取表单数据
var name = document.getElementById("name").value;
var email = document.getElementById("email").value;
var comment = document.getElementById("comment").value;
// 拼接评论html代码
var commentHtml = "<div class='comment-section'>";
commentHtml += "<h3>" + name + "</h3>";
commentHtml += "<p>" + comment + "</p>";
commentHtml += "<p>" + email + "</p>";
commentHtml += "</div>";
// 将评论添加到页面中
var comments = document.getElementsByClassName("comment-section");
if (comments.length > 0) {
comments[0].insertAdjacentHTML('afterend', commentHtml);
} else {
document.body.insertAdjacentHTML('beforeend', commentHtml);
}
// 清空表单数据
document.getElementById("name").value = "";
document.getElementById("email").value = "";
document.getElementById("comment").value = "";
}
</script>
</body>
</html>
阅读全文
相关推荐
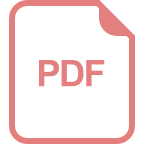

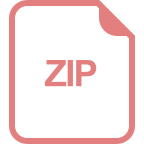
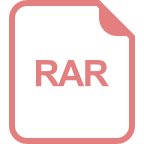
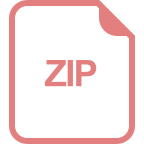
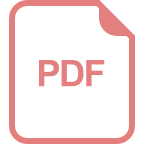
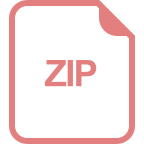
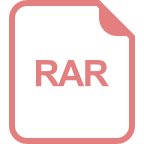
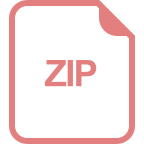
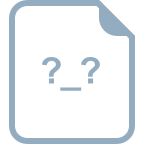
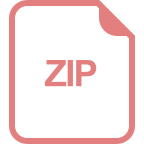
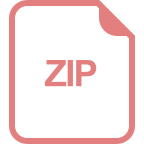
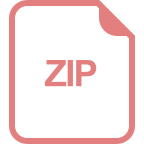
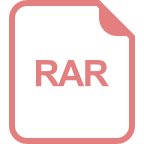
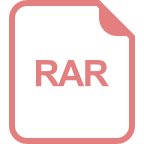
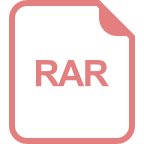
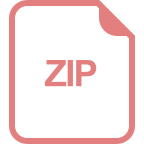