linux系统下使用ffmpeg代码解码h264实时视频
时间: 2023-11-14 09:10:06 浏览: 54
在 Linux 系统下使用 FFmpeg 解码 H.264 实时视频,可以按照以下步骤进行:
1. 安装 FFmpeg
首先需要安装 FFmpeg 库,可以使用以下命令在 Ubuntu 系统中安装:
```
sudo apt-get install ffmpeg
```
2. 编写代码
接下来需要编写 C++ 代码,使用 FFmpeg 库进行 H.264 视频解码。
示例代码如下:
```c++
#include <iostream>
#include <fstream>
#include <string.h>
#include <stdio.h>
extern "C"
{
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
#include <libavutil/imgutils.h>
}
using namespace std;
int main(int argc, char* argv[])
{
AVFormatContext* pFormatCtx = NULL;
AVCodecContext* pCodecCtx = NULL;
AVCodec* pCodec = NULL;
AVFrame* pFrame = NULL;
AVFrame* pFrameRGB = NULL;
uint8_t* buffer = NULL;
int numBytes;
if(argc < 2)
{
printf("Please provide a H264 video file.\n");
return -1;
}
av_register_all();
if(avformat_open_input(&pFormatCtx, argv[1], NULL, NULL) != 0)
{
printf("Error: Cannot open video file.\n");
return -1;
}
if(avformat_find_stream_info(pFormatCtx, NULL) < 0)
{
printf("Error: Cannot find stream information.\n");
return -1;
}
AVCodecParameters* pCodecParams = NULL;
int videoStreamIndex = -1;
for(int i = 0; i < pFormatCtx->nb_streams; i++)
{
if(pFormatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO)
{
videoStreamIndex = i;
pCodecParams = pFormatCtx->streams[i]->codecpar;
break;
}
}
if(videoStreamIndex == -1)
{
printf("Error: Cannot find video stream.\n");
return -1;
}
pCodec = avcodec_find_decoder(pCodecParams->codec_id);
if(pCodec == NULL)
{
printf("Error: Cannot find codec.\n");
return -1;
}
pCodecCtx = avcodec_alloc_context3(pCodec);
if(avcodec_parameters_to_context(pCodecCtx, pCodecParams) != 0)
{
printf("Error: Cannot copy codec parameters to context.\n");
return -1;
}
if(avcodec_open2(pCodecCtx, pCodec, NULL) < 0)
{
printf("Error: Cannot open codec.\n");
return -1;
}
pFrame = av_frame_alloc();
pFrameRGB = av_frame_alloc();
if(pFrame == NULL || pFrameRGB == NULL)
{
printf("Error: Cannot allocate frames.\n");
return -1;
}
numBytes = av_image_get_buffer_size(AV_PIX_FMT_RGB24, pCodecCtx->width, pCodecCtx->height, 1);
buffer = (uint8_t*)av_malloc(numBytes * sizeof(uint8_t));
av_image_fill_arrays(pFrameRGB->data, pFrameRGB->linesize, buffer, AV_PIX_FMT_RGB24, pCodecCtx->width, pCodecCtx->height, 1);
AVPacket packet;
int frameFinished = 0;
struct SwsContext* sws_ctx = NULL;
while(av_read_frame(pFormatCtx, &packet) >= 0)
{
if(packet.stream_index == videoStreamIndex)
{
avcodec_send_packet(pCodecCtx, &packet);
while(avcodec_receive_frame(pCodecCtx, pFrame) == 0)
{
sws_ctx = sws_getContext(pCodecCtx->width, pCodecCtx->height, pCodecCtx->pix_fmt, pCodecCtx->width, pCodecCtx->height, AV_PIX_FMT_RGB24, SWS_BILINEAR, NULL, NULL, NULL);
sws_scale(sws_ctx, pFrame->data, pFrame->linesize, 0, pCodecCtx->height, pFrameRGB->data, pFrameRGB->linesize);
sws_freeContext(sws_ctx);
printf("Decoding frame %d\n", pCodecCtx->frame_number);
}
}
av_packet_unref(&packet);
}
av_free(buffer);
av_free(pFrameRGB);
av_free(pFrame);
avcodec_close(pCodecCtx);
avformat_close_input(&pFormatCtx);
return 0;
}
```
3. 编译代码
使用以下命令编译上述代码:
```
g++ -o decode_h264 decode_h264.cpp -lavformat -lavcodec -lswscale -lavutil
```
4. 运行代码
使用以下命令运行编译好的代码:
```
./decode_h264 input.h264
```
其中 `input.h264` 是待解码的 H.264 视频文件名。
以上就是在 Linux 系统下使用 FFmpeg 解码 H.264 实时视频的过程。
相关推荐
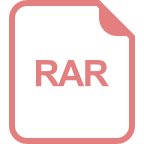
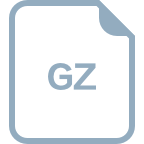
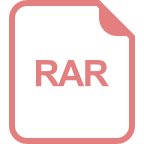














