用mind+软件 创建链表score,存储10个数字,找出最大值
时间: 2024-09-10 10:20:00 浏览: 24
在Mind+软件中创建一个链表并存储10个数字,首先需要定义链表节点结构,包含一个数值变量和指向下一个节点的指针。以下是简单的步骤:
1. **创建数据结构**:
- 设计一个名为`ScoreNode`的节点类,它有一个属性如`value`表示分数,并有`next`属性作为链接到下一个节点的引用。
```python
class ScoreNode:
def __init__(self, score=0):
self.value = score
self.next = None
```
2. **初始化链表**:
- 创建一个空链表`head`,用于保存第一个节点。
```python
def create_linked_list():
head = ScoreNode()
current = head
for i in range(10):
current.next = ScoreNode(i + 1) # 假设从1开始计数
current = current.next
return head
```
3. **查找最大值**:
- 定义一个函数来遍历链表并找到最大值。
```python
def find_max_value(head):
max_score = head.value
current = head
while current is not None:
if current.value > max_score:
max_score = current.value
current = current.next
return max_score
```
4. **整合流程**:
- 首先创建链表,然后调用`find_max_value`函数找出最大值。
```python
if __name__ == "__main__":
head = create_linked_list()
max_score = find_max_value(head)
print(f"链表中的最大值是: {max_score}")
```
完成以上步骤后,你可以运行上述代码,在Mind+环境中看到链表的创建和最大值的寻找结果。
相关推荐


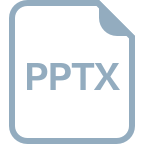
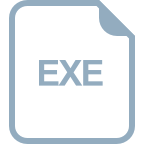
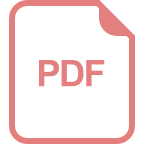
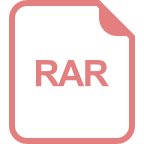
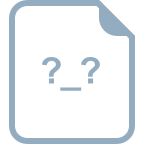
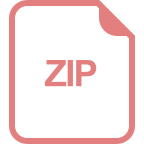
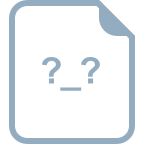


