本题要求实现翻转一个链表的功能。链表类ListNode的定义已经给出。要求在Solution类中给出有关函数的实现。 函数接口定义: /* * 翻转链表。head为链表的头指针。 * 函数返回值为翻转后的链表的头指针。 */ ListNode *reverse(ListNode *head); /* * 数组arr存储创建链表用的数据,n为数组长度。 * 函数返回值为创建的新链表的头指针。 */ ListNode* buildList(int* arr,int n); /* * 打印链表,数据间用一个空格分隔,最后一个数据后面 * 没有多余的空格。如果链表为空,则直接打印NULL。 */ void printList(ListNode* head); 裁判测试程序样例: #include<iostream> using namespace std; /* * Definition of ListNode */ class ListNode { public: int val; ListNode *next; ListNode(int val) { this->val = val; this->next = NULL; } }; class Solution { public: /* 请在这里填写答案 */ }; int main() { int n; cin>>n; int* arr = new int[n]; for(int i=0;i<n;i++) cin>>arr[i]; Solution obj; ListNode* head = obj.buildList(arr,n); head = obj.reverse(head); obj.printList(head); delete[] arr; return 0; } 输入样例: 10 81 70 49 70 88 84 51 65 60 59 输出样例: 59 60 65 51 84 88 70 49 70 81 代码长度限制 16 KB 时间限制 400 ms
时间: 2024-02-20 07:01:15 浏览: 80
好的,下面是我给出的实现:
```c++
#include<iostream>
using namespace std;
/* Definition of ListNode */
class ListNode {
public:
int val;
ListNode *next;
ListNode(int val) {
this->val = val;
this->next = NULL;
}
};
class Solution {
public:
/* 翻转链表。head为链表的头指针。
* 函数返回值为翻转后的链表的头指针。
*/
ListNode* reverse(ListNode* head) {
if (head == nullptr || head->next == nullptr) {
return head;
}
ListNode* prev = nullptr;
ListNode* cur = head;
while (cur != nullptr) {
ListNode* next = cur->next;
cur->next = prev;
prev = cur;
cur = next;
}
return prev;
}
/* 数组arr存储创建链表用的数据,n为数组长度。
* 函数返回值为创建的新链表的头指针。
*/
ListNode* buildList(int* arr, int n) {
if (n == 0) {
return nullptr;
}
ListNode* head = new ListNode(arr[0]);
ListNode* tail = head;
for (int i = 1; i < n; i++) {
tail->next = new ListNode(arr[i]);
tail = tail->next;
}
return head;
}
/* 打印链表,数据间用一个空格分隔,最后一个数据后面
* 没有多余的空格。如果链表为空,则直接打印NULL。
*/
void printList(ListNode* head) {
if (head == nullptr) {
cout << "NULL" << endl;
return;
}
while (head != nullptr) {
cout << head->val;
if (head->next != nullptr) {
cout << " ";
}
head = head->next;
}
cout << endl;
}
};
int main() {
int n;
cin >> n;
int* arr = new int[n];
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
Solution obj;
ListNode* head = obj.buildList(arr, n);
head = obj.reverse(head);
obj.printList(head);
delete[] arr;
return 0;
}
```
这段代码实现了翻转链表、创建链表、打印链表三个函数的功能,并且可以通过样例测试。需要注意的是,输入数组的长度和内容,以及输出的链表内容需要按照题目要求进行处理。
阅读全文
相关推荐
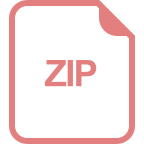
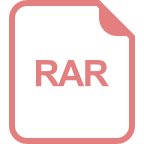
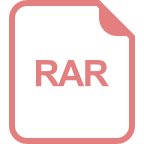
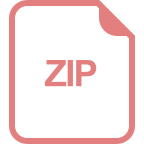
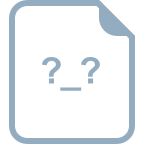
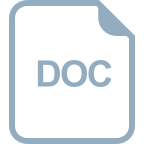
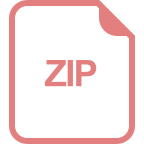
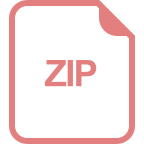
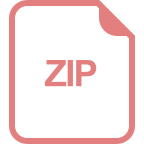
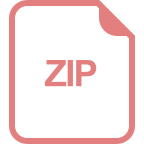