google.protobuf.BytesValue 如何赋值
时间: 2023-07-07 18:35:06 浏览: 53
你可以通过以下方式对 `google.protobuf.BytesValue` 类型进行赋值:
```python
import google.protobuf
# 创建一个 BytesValue 对象
bytes_value = google.protobuf.wrappers_pb2.BytesValue()
# 对 BytesValue 对象赋值
bytes_value.value = b'your bytes value here'
```
在上面的代码中,我们首先导入了 `google.protobuf` 模块,然后创建了一个空的 `BytesValue` 对象。接着,我们可以通过 `bytes_value.value` 属性对这个对象进行赋值,其中 `b'your bytes value here'` 是你要赋的值,需要用字节字符串的形式表示。
相关问题
google.protobuf.BytesValue
`google.protobuf.BytesValue` 是 Google Protocol Buffer 中的一个消息类型,它表示一个字节数组。下面是一个 `google.protobuf.BytesValue` 的示例:
```protobuf
syntax = "proto3";
import "google/protobuf/wrappers.proto";
message MyMessage {
google.protobuf.BytesValue my_field = 1;
}
```
在这个示例中,我们定义了一个名为 `MyMessage` 的消息类型,它包含一个名为 `my_field` 的字段,类型为 `google.protobuf.BytesValue`。这个字段可以存储任意长度的字节数组。
如果你想要设置 `my_field` 字段的值,可以使用以下代码:
```python
from google.protobuf import bytes_pb2
my_message = MyMessage()
my_message.my_field = bytes_pb2.BytesValue(value=b'hello world')
```
在这个示例中,我们首先创建了一个 `MyMessage` 的实例 `my_message`,然后将 `my_field` 字段设置为一个包含字符串 `'hello world'` 的字节数组。
如果你想要获取 `my_field` 字段的值,可以使用以下代码:
```python
my_bytes = my_message.my_field.value
```
在这个示例中,我们首先获取了 `my_field` 字段的值,然后将其赋值给变量 `my_bytes`。
C++ google.protobuf.BytesValue
C++中的google.protobuf.BytesValue是一个用于存储字节数据的类。它是Google Protocol Buffers库中的一部分,用于在消息中存储二进制数据。下面是一个使用google.protobuf.BytesValue的示例:
```cpp
#include <iostream>
#include <string>
#include <google/protobuf/any.pb.h>
int main() {
google::protobuf::BytesValue bytes_value;
std::string data = "Hello, World!";
bytes_value.set_value(data);
std::cout << "BytesValue: " << bytes_value.value() << std::endl;
return 0;
}
```
在这个示例中,我们首先包含了google/protobuf/any.pb.h头文件,该头文件包含了google.protobuf.BytesValue类的定义。然后,我们创建了一个BytesValue对象,并使用set_value()方法将字符串数据设置为BytesValue的值。最后,我们使用value()方法获取BytesValue的值并打印出来。
相关推荐
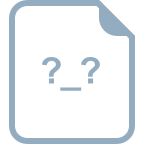
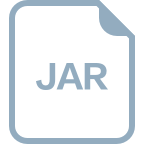












