编写一个C语言代码程序,设计一种好的数据结构,尽可能高效地实现元素的插入、删除、按值查找和按序号查找(假设所有元素值不同)
时间: 2023-03-30 10:01:25 浏览: 87
好的,我可以回答这个问题。以下是一个示例代码,使用链表实现数据结构,可以高效地实现元素的插入、删除、按值查找和按序号查找:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct node {
int value;
struct node *next;
} Node;
// 定义链表结构体
typedef struct {
Node *head;
int length;
} List;
// 初始化链表
void initList(List *list) {
list->head = NULL;
list->length = ;
}
// 在链表末尾插入元素
void insert(List *list, int value) {
Node *newNode = (Node*) malloc(sizeof(Node));
newNode->value = value;
newNode->next = NULL;
if (list->head == NULL) {
list->head = newNode;
} else {
Node *p = list->head;
while (p->next != NULL) {
p = p->next;
}
p->next = newNode;
}
list->length++;
}
// 删除链表中的元素
void delete(List *list, int value) {
Node *p = list->head;
Node *prev = NULL;
while (p != NULL) {
if (p->value == value) {
if (prev == NULL) {
list->head = p->next;
} else {
prev->next = p->next;
}
free(p);
list->length--;
return;
}
prev = p;
p = p->next;
}
}
// 按值查找链表中的元素
int searchByValue(List *list, int value) {
Node *p = list->head;
while (p != NULL) {
if (p->value == value) {
return 1;
}
p = p->next;
}
return ;
}
// 按序号查找链表中的元素
int searchByIndex(List *list, int index) {
if (index < || index >= list->length) {
return -1;
}
Node *p = list->head;
int i = ;
while (p != NULL) {
if (i == index) {
return p->value;
}
i++;
p = p->next;
}
return -1;
}
int main() {
List list;
initList(&list);
insert(&list, 1);
insert(&list, 2);
insert(&list, 3);
printf("List length: %d\n", list.length);
delete(&list, 2);
printf("List length: %d\n", list.length);
printf("Search by value: %d\n", searchByValue(&list, 3));
printf("Search by index: %d\n", searchByIndex(&list, 1));
return ;
}
```
相关推荐
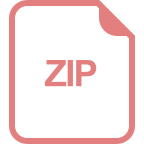














