C#如何调用qt写的窗口
时间: 2023-10-09 17:07:51 浏览: 82
如果您想在 C# 中使用 Qt 编写的窗口,可以使用 Qt 的 C++ 接口(即 Qt 的跨语言支持),并将其封装为 C++/CLI 类型,以便在 C# 中调用。以下是实现步骤:
1. 首先,在 Qt 中编写您的窗口并在 C++ 程序中进行测试。
2. 然后,将您的 Qt 代码编译为动态链接库(DLL)或静态链接库(LIB)。
3. 接下来,使用 C++/CLI 编写一个中间层,该层将 Qt 代码封装为可从 C# 中调用的类型。
4. 最后,在 C# 代码中实例化该中间层,并使用它来调用 Qt 窗口。
下面是一个简单的示例,说明如何在 C# 中调用 Qt 窗口:
1. 创建一个 Qt 应用程序,并将其编译为 DLL 文件。
2. 在 C++/CLI 中创建一个类,该类包装 Qt 窗口,并公开 C# 可调用的方法。
```cpp
#include "myqtwindow.h"
using namespace System;
using namespace System::Runtime::InteropServices;
namespace MyWrapper {
public ref class MyQtWindowWrapper {
private:
MyQtWindow* m_pWindow;
public:
MyQtWindowWrapper() {
m_pWindow = new MyQtWindow();
}
~MyQtWindowWrapper() {
delete m_pWindow;
}
void Show() {
m_pWindow->show();
}
void SetTitle(String^ title) {
const char* str = (const char*)(Marshal::StringToHGlobalAnsi(title)).ToPointer();
m_pWindow->setWindowTitle(str);
Marshal::FreeHGlobal(IntPtr((void*)str));
}
// Add more methods here as needed
};
}
```
3. 在 C# 中实例化 MyQtWindowWrapper 类,并使用它来调用 Qt 窗口。
```csharp
using System;
using MyWrapper;
namespace MyApplication {
class Program {
static void Main(string[] args) {
MyQtWindowWrapper wrapper = new MyQtWindowWrapper();
wrapper.SetTitle("My Qt Window");
wrapper.Show();
}
}
}
```
请注意,这只是一个简单的示例,您需要根据您的具体情况进行调整。
相关推荐
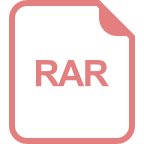
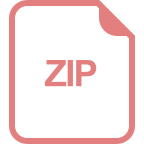
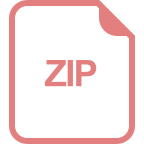
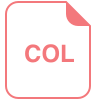
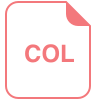
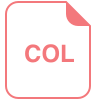
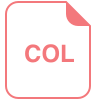
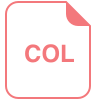









