java clickhouse 每15分钟批量插入一年的随机数据
时间: 2023-12-10 10:36:53 浏览: 114
首先,需要使用Java ClickHouse客户端连接到ClickHouse数据库。然后,可以使用Java的定时任务功能(例如ScheduledExecutorService)每15分钟执行一次插入操作。
以下是一个简单的示例代码:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Random;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class ClickHouseBatchInsert {
private static final String CLICKHOUSE_URL = "jdbc:clickhouse://localhost:8123/default";
private static final String CLICKHOUSE_USER = "default";
private static final String CLICKHOUSE_PASSWORD = "";
private static final String INSERT_SQL = "INSERT INTO my_table (timestamp, value) VALUES (?, ?)";
private static final int BATCH_SIZE = 10000;
private static final int YEAR = 2022;
private static final Random RANDOM = new Random();
public static void main(String[] args) throws SQLException {
Connection connection = DriverManager.getConnection(CLICKHOUSE_URL, CLICKHOUSE_USER, CLICKHOUSE_PASSWORD);
ScheduledExecutorService executor = Executors.newSingleThreadScheduledExecutor();
executor.scheduleAtFixedRate(() -> {
try {
insertDataForYear(connection, YEAR);
} catch (SQLException e) {
e.printStackTrace();
}
}, 0, 15, TimeUnit.MINUTES);
}
private static void insertDataForYear(Connection connection, int year) throws SQLException {
PreparedStatement stmt = connection.prepareStatement(INSERT_SQL);
for (int month = 1; month <= 12; month++) {
for (int dayOfMonth = 1; dayOfMonth <= 31; dayOfMonth++) {
for (int hour = 0; hour <= 23; hour++) {
for (int minute = 0; minute <= 59; minute++) {
long timestamp = getTimestamp(year, month, dayOfMonth, hour, minute);
double value = RANDOM.nextDouble();
stmt.setLong(1, timestamp);
stmt.setDouble(2, value);
stmt.addBatch();
if (stmt.getBatchSize() >= BATCH_SIZE) {
stmt.executeBatch();
}
}
}
}
}
stmt.executeBatch();
stmt.close();
}
private static long getTimestamp(int year, int month, int dayOfMonth, int hour, int minute) {
return java.time.LocalDateTime.of(year, month, dayOfMonth, hour, minute).atZone(java.time.ZoneId.systemDefault()).toInstant().toEpochMilli();
}
}
```
在上面的示例代码中,我们使用了Java 8的LocalDateTime和ZoneId来生成时间戳。如果您使用的是Java 7或更早版本,则需要使用Java的Calendar类来生成时间戳。
此外,注意我们将每批次插入的大小设置为10000。这可以根据您的具体情况进行调整,以获得最佳性能。
阅读全文
相关推荐
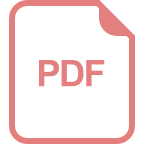
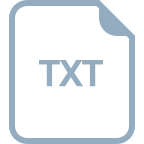
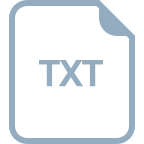
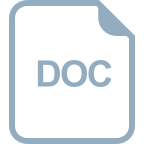
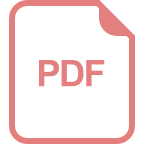
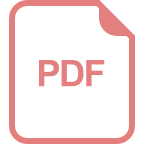
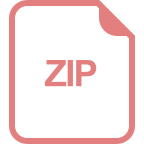
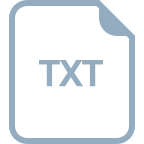
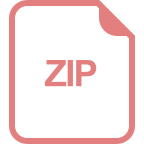
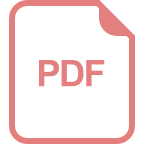
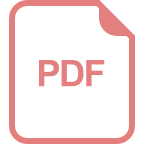
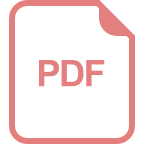
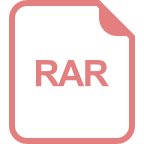
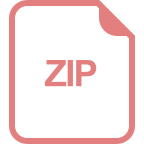
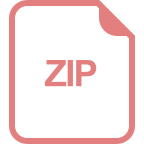