用c++多组输入一行文字,并统计文字中空格的个数并输出
时间: 2023-06-17 20:04:35 浏览: 99
下面是一份简单的 C++ 代码示例,可以读取多组输入,并统计输入行中空格的数量:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
string line;
while (getline(cin, line)) {
int count = 0;
for (int i = 0; i < line.length(); i++) {
if (line[i] == ' ') {
count++;
}
}
cout << "空格的个数为:" << count << endl;
}
return 0;
}
```
在这个示例中,我们使用 `getline()` 函数读取每一行输入,并将其存储为一个字符串变量 `line`。然后使用一个循环遍历字符串中的每个字符,如果发现某个字符是空格,则将计数器 `count` 的值加一。最后输出统计结果。这段代码可以处理多组输入,直到用户输入结束符为止。
相关问题
用c++语音,统计一行文字中空格的个数并输出,数据多组输入
以下是用 C++ 编写的程序,可以统计一行文字中空格的个数并输出,支持多组数据输入:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string line;
while (getline(cin, line)) {
int count = 0;
for (char c : line) {
if (c == ' ') {
count++;
}
}
cout << count << endl;
}
return 0;
}
```
程序中使用了一个 `while` 循环,不断读入输入的每一行文字。对于每一行文字,使用一个循环遍历每个字符,如果该字符是空格,则空格计数器加一。最后输出空格计数器的值。
需要注意的是,程序中使用了 `getline` 函数来读入一行文字。该函数会读入一行文字并返回一个 `string` 类型的对象,因此需要包含 `<string>` 头文件。另外,由于输入包含多组数据,因此需要使用一个 `while` 循环不断读入输入,直到读到文件结尾或输入结束符为止。
用c++统计一行文字中空格的个数并输出。
可以使用循环遍历字符串的每个字符,判断是否为空格,如果是则计数器加1。以下是一个简单的示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string text = "Hello world, how are you?";
int count = 0;
for (int i = 0; i < text.length(); i++) {
if (text[i] == ' ') {
count++;
}
}
cout << "Number of spaces in text: " << count << endl;
return 0;
}
```
输出结果为:
```
Number of spaces in text: 5
```
阅读全文
相关推荐
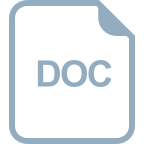
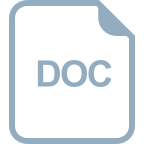
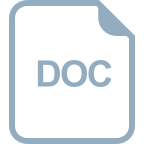













