写一段python代码,使用opencv检测apriltag的姿态
时间: 2024-09-08 08:00:58 浏览: 53
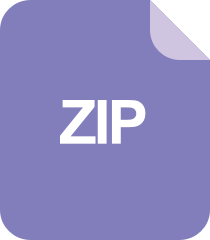
使用摄像头实时人脸检测 Python代码 基于OpenCV和RetinaFace

在Python中,可以使用OpenCV库中的函数来检测apriltag的姿态。首先确保你已经安装了`opencv-python`和`opencv-contrib-python`,因为apriltag模块是OpenCV的一个扩展模块。以下是一个简单的代码示例,演示了如何使用OpenCV检测apriltag,并获取其姿态估计。
```python
import cv2
import numpy as np
# 加载apriltag检测器
tagFamily = 'tag36h11'
detector = cv2.AprilTagDetector_create(tagFamily=tagFamily)
# 读取图像
image_path = 'path_to_your_image.jpg' # 替换为你的图片路径
img = cv2.imread(image_path)
# 转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测apriltag
tags, _ = detector.detect(gray)
# 输出apriltag的数量
print(f"Detected {len(tags)} tag(s)")
# 如果检测到apriltag,绘制边界框和姿态
for tag in tags:
# 绘制边界框
pt1 = tag.get_first_left_pair()
pt2 = tag.get_second_left_pair()
cv2.line(img, pt1, pt2, (0, 255, 0), 3)
# 获取并打印姿态信息
tag_pose, tag_error = tag.get_pose(tagSize=1.0)
print(f"Tag ID: {tag.id}, Pose: {tag_pose}")
# 绘制姿态轴(如果需要)
cv2.drawFrameAxes(img, None, None, tag_pose, tag_error, length=100)
# 显示图像
cv2.imshow('AprilTag Detection', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这段代码中,我们首先创建了一个apriltag检测器对象,然后读取一张图片并将其转换为灰度图像。接下来使用`detect`方法检测图像中的apriltag,并对每个检测到的apriltag绘制边界框和姿态轴。
请确保替换`path_to_your_image.jpg`为实际的图片路径,并且根据实际情况调整`tagSize`参数以适应你的apriltag的实际尺寸。
阅读全文
相关推荐
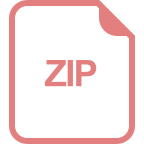
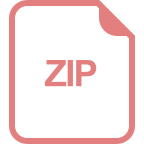
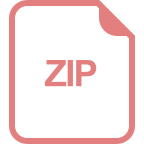
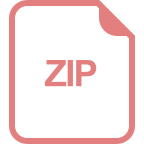
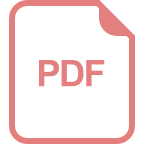
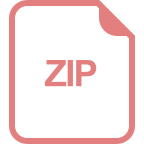
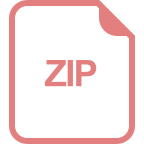
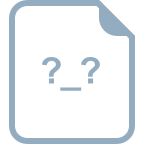
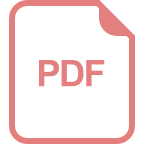
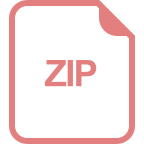
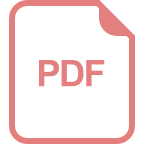
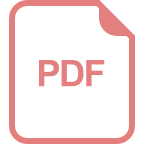
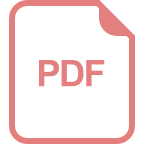
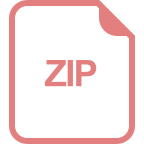
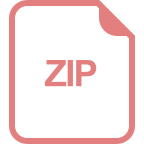
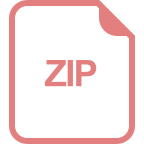