基于eclipse和sql的管理系统代码
时间: 2023-07-31 19:02:02 浏览: 136
基于Eclipse和SQL的管理系统代码可以使用Java编程语言编写。以下是一个示例代码,用于演示如何使用Eclipse和SQL来创建一个简单的管理系统。
首先,你需要建立一个连接到数据库的类,用于连接和执行SQL查询。我们可以使用JDBC(Java数据库连接)来实现这个类。
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnector {
private Connection connection;
public Connection getConnection() {
return connection;
}
public void connect(String url, String username, String password) throws SQLException {
connection = DriverManager.getConnection(url, username, password);
}
public void disconnect() throws SQLException {
if (connection != null) {
connection.close();
}
}
}
```
接下来,我们可以创建一个管理系统类,用于执行各种操作。例如,我们可以创建一个学生管理系统,用于添加、删除和查询学生信息。
```java
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class StudentManagementSystem {
private DatabaseConnector connector;
public StudentManagementSystem(DatabaseConnector connector) {
this.connector = connector;
}
public void addStudent(String name, int age) throws SQLException {
Connection connection = connector.getConnection();
String sql = "INSERT INTO students (name, age) VALUES (?, ?)";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, name);
statement.setInt(2, age);
statement.executeUpdate();
statement.close();
}
public void deleteStudent(int studentId) throws SQLException {
Connection connection = connector.getConnection();
String sql = "DELETE FROM students WHERE id = ?";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setInt(1, studentId);
statement.executeUpdate();
statement.close();
}
public void queryStudent(int studentId) throws SQLException {
Connection connection = connector.getConnection();
String sql = "SELECT * FROM students WHERE id = ?";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setInt(1, studentId);
ResultSet resultSet = statement.executeQuery();
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
int age = resultSet.getInt("age");
System.out.println("ID: " + id + ", Name: " + name + ", Age: " + age);
}
resultSet.close();
statement.close();
}
}
```
最后,在主程序中,我们可以创建一个DatabaseConnector对象,建立与数据库的连接,然后使用这个连接来执行各种操作。
```java
public class Main {
public static void main(String[] args) {
// 创建数据库连接器
DatabaseConnector connector = new DatabaseConnector();
try {
// 连接到数据库
connector.connect("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
// 创建管理系统对象
StudentManagementSystem system = new StudentManagementSystem(connector);
// 添加学生
system.addStudent("张三", 18);
// 删除学生
system.deleteStudent(1);
// 查询学生
system.queryStudent(2);
// 断开与数据库的连接
connector.disconnect();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
以上代码演示了如何使用Eclipse和SQL来创建一个基本的管理系统。当然,实际的管理系统会更复杂,具体的实现和功能取决于你的需求。这只是一个简单的示例用于说明基本的开发过程。
阅读全文
相关推荐
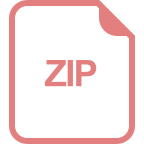
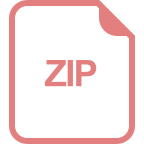
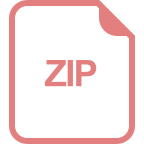
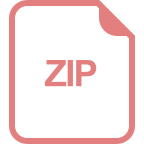
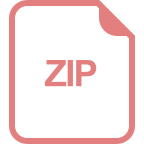
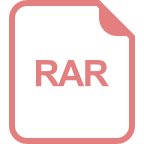
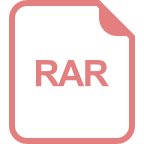
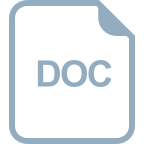
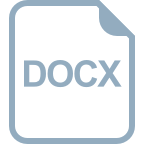
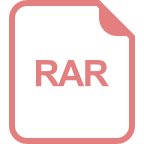
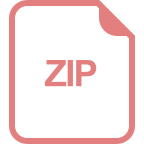
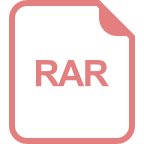
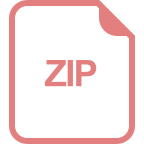
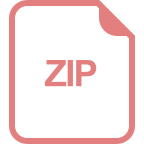
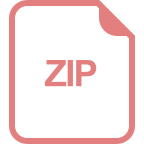
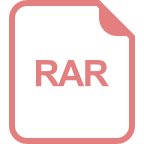

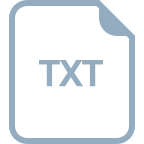