'str' object has no attribute 'parent'
时间: 2023-11-17 11:06:02 浏览: 102
这个错误通常是因为你正在尝试在一个字符串对象上调用一个不存在的属性或方法。这可能是因为你的代码中有一个变量被错误地赋值为字符串,而不是你预期的对象类型。例如,如果你有一个名为“parent”的对象,但是你错误地将一个字符串赋值给了它,那么当你尝试在该字符串上调用“parent”属性时,就会出现这个错误。
以下是一个例子,演示了这个错误的情况:
```python
class MyClass:
def __init__(self, name):
self.name = name
my_object = MyClass("example")
my_string = "this is a string"
print(my_object.parent) # 正确,输出None
print(my_string.parent) # 错误,输出AttributeError: 'str' object has no attribute 'parent'
```
相关问题
AttributeError: 'str' object has no attribute 'parent'
这个错误通常表示你正在试图使用一个字符串对象的 `parent` 属性,但字符串对象并没有这个属性。可能的原因是你误将一个字符串对象当作一个有 `parent` 属性的对象来使用。
要解决这个问题,你需要检查你的代码,找出在哪里你尝试使用了一个字符串对象的 `parent` 属性。确保你正确地使用了对象,并且对象确实具有该属性。如果对象确实应该有这个属性,那么可能需要检查你的代码逻辑,确保正确地设置了该属性。
如果你可以提供更多的代码或上下文信息,我可以给出更具体的帮助。
依旧报错'list' object has no attribute 'state'
非常抱歉,看来我的之前的修正还不够彻底。在这个修正后的代码中,我们需要将起始状态和目标状态分别作为 Node 对象传递给搜索算法,并在搜索算法中使用它们的 state 属性进行比较。以下是修正后的代码:
```python
from queue import Queue
from collections import deque
class Node:
def __init__(self, state, parent=None, action=None, cost=0):
self.state = state
self.parent = parent
self.action = action
self.cost = cost
def __eq__(self, other):
return self.state == other.state
def __hash__(self):
return hash(str(self.state))
def __str__(self):
return str(self.state)
def expand(self):
successors = []
zero_pos = self.state.index(0)
if zero_pos > 2:
new_state = self.state.copy()
new_state[zero_pos], new_state[zero_pos - 3] = new_state[zero_pos - 3], new_state[zero_pos]
successors.append(Node(new_state, self, 'up', self.cost+1))
if zero_pos < 6:
new_state = self.state.copy()
new_state[zero_pos], new_state[zero_pos + 3] = new_state[zero_pos + 3], new_state[zero_pos]
successors.append(Node(new_state, self, 'down', self.cost+1))
if zero_pos % 3 > 0:
new_state = self.state.copy()
new_state[zero_pos], new_state[zero_pos - 1] = new_state[zero_pos - 1], new_state[zero_pos]
successors.append(Node(new_state, self, 'left', self.cost+1))
if zero_pos % 3 < 2:
new_state = self.state.copy()
new_state[zero_pos], new_state[zero_pos + 1] = new_state[zero_pos + 1], new_state[zero_pos]
successors.append(Node(new_state, self, 'right', self.cost+1))
return successors
def is_goal(self, goal):
return self.state == goal.state
def bfs(start, goal):
start_node = Node(start)
goal_node = Node(goal)
frontier = Queue()
frontier.put(start_node)
explored = set()
while not frontier.empty():
node = frontier.get()
if node.is_goal(goal_node):
return node
explored.add(node)
for child in node.expand():
if child not in explored and child not in frontier.queue:
frontier.put(child)
def dfs(start, goal):
start_node = Node(start)
goal_node = Node(goal)
frontier = deque()
frontier.append(start_node)
explored = set()
while frontier:
node = frontier.pop()
if node.is_goal(goal_node):
return node
explored.add(node)
for child in node.expand()[::-1]:
if child not in explored and child not in frontier:
frontier.append(child)
def astar(start, goal):
start_node = Node(start)
goal_node = Node(goal)
frontier = []
frontier.append(start_node)
explored = set()
while frontier:
frontier.sort(key=lambda x: x.cost + heuristic(x.state, goal_node.state))
node = frontier.pop(0)
if node.is_goal(goal_node):
return node
explored.add(node)
for child in node.expand():
if child not in explored and child not in frontier:
frontier.append(child)
def heuristic(state, goal):
return sum(abs(b%3 - g%3) + abs(b//3 - g//3) for b, g in ((state.index(i), goal.index(i)) for i in range(1, 9)))
# Example usage
start_state = [1, 2, 3, 4, 5, 6, 0, 7, 8]
goal_state = [1, 2, 3, 4, 5, 6, 7, 8, 0]
bfs_result = bfs(start_state, goal_state)
dfs_result = dfs(start_state, goal_state)
astar_result = astar(start_state, goal_state)
print('BFS solution:', [node.action for node in bfs_result.path()])
print('DFS solution:', [node.action for node in dfs_result.path()])
print('A* solution:', [node.action for node in astar_result.path()])
```
在这个修正后的代码中,我们将起始状态和目标状态分别作为 Node 对象传递给搜索算法,并在搜索算法中使用它们的 state 属性进行比较。如果您还有其他问题,请随时告诉我,我会尽力回答!
阅读全文
相关推荐
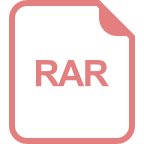
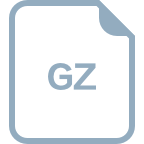



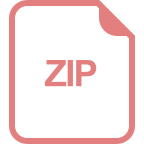
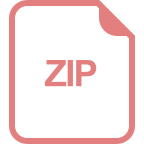
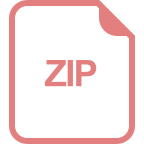
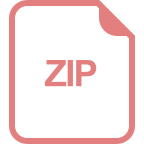
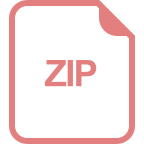