定义一个数组,其中包含产品的名称、单价和购买数量,使用methods(方法)、computed(计算属性)和 watch(侦听器)三者中最优的方式计算购物车中产品的总价格;然后修改产品的购买数量,并重新计算其总价格写出完整代码
时间: 2024-10-07 11:00:52 浏览: 58
在Vue.js中,我们可以使用`data`对象来初始化产品数据,然后通过`methods`来定义计算总价的方法,利用`computed`来创建响应式的价格计算属性,以及`watch`来监听数量变化并自动更新总价。这是一种常见的优化方式,因为它将计算逻辑分离到了单独的地方,保持了视图层的简洁。
首先,定义数据结构:
```javascript
export default {
data() {
return {
products: [
{ name: 'Product 1', price: 10.99, quantity: 2 },
{ name: 'Product 2', price: 5.49, quantity: 5 }
]
};
},
};
```
接下来,在`methods`中定义计算总价的方法:
```javascript
methods: {
calculateTotalPrice(product) {
return product.price * product.quantity;
},
},
```
使用`computed`创建响应式的总价属性:
```javascript
computed: {
totalPrice() {
let total = 0;
this.products.forEach(product => {
total += this.calculateTotalPrice(product);
});
return total;
}
}
```
最后,使用`watch`监听数量变化:
```javascript
watch: {
'products.*.quantity': function (newQuantity, oldQuantity) {
this.totalPrice = this.calculateTotalPrice(this.products.find(product => product.name === this.$options.name)); // 假设产品名已绑定到组件实例上
}
}
```
这样,当产品数量发生变化时,总价会自动重新计算。
完整代码示例:
```javascript
<template>
<ul>
<li v-for="product in products" :key="product.name">
{{ product.name }} - Price: {{ product.price }}, Quantity: {{ product.quantity }}
<!-- Total Price will be displayed here -->
</li>
</ul>
</template>
<script>
export default {
data() {
return {
products: [
{ name: 'Product 1', price: 10.99, quantity: 2 },
{ name: 'Product 2', price: 5.49, quantity: 5 }
]
};
},
methods: {
calculateTotalPrice(product) {
return product.price * product.quantity;
}
},
computed: {
totalPrice() {
let total = 0;
this.products.forEach(product => {
total += this.calculateTotalPrice(product);
});
return total;
}
},
watch: {
'products.*.quantity': function (newQuantity, oldQuantity) {
this.totalPrice = this.calculateTotalPrice(this.products.find(product => product.name === this.$options.name));
}
}
};
</script>
```
阅读全文
相关推荐
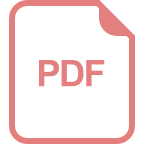
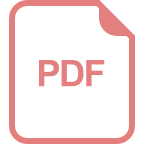
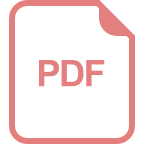










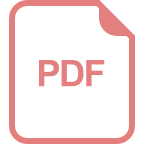



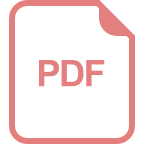