帕恰狗python代码
时间: 2023-11-09 15:00:51 浏览: 89
以下是帕恰狗的 Python 代码:
```python
import random
class PachaDog:
def __init__(self, name):
self.name = name
self.tricks = []
def teach_trick(self, trick):
self.tricks.append(trick)
def do_trick(self):
if self.tricks:
trick = random.choice(self.tricks)
print(f"{self.name} does a {trick}!")
else:
print(f"{self.name} doesn't know any tricks yet.")
pacha = PachaDog("Pacha")
pacha.teach_trick("sit")
pacha.teach_trick("roll over")
pacha.teach_trick("play dead")
pacha.do_trick()
pacha.do_trick()
pacha.do_trick()
```
输出:
```
Pacha does a sit!
Pacha does a play dead!
Pacha does a roll over!
```
相关问题
画帕恰狗python代码
以下是画帕恰狗的 Python 代码:
```python
import turtle
# 画头
turtle.penup()
turtle.goto(0, -100)
turtle.pendown()
turtle.circle(100)
# 画左耳朵
turtle.penup()
turtle.goto(-70, 40)
turtle.pendown()
turtle.setheading(-60)
turtle.circle(80, 120)
# 画右耳朵
turtle.penup()
turtle.goto(70, 40)
turtle.pendown()
turtle.setheading(60)
turtle.circle(-80, 120)
# 画左眼
turtle.penup()
turtle.goto(-40, 120)
turtle.pendown()
turtle.fillcolor("white")
turtle.begin_fill()
turtle.circle(20)
turtle.end_fill()
# 画右眼
turtle.penup()
turtle.goto(40, 120)
turtle.pendown()
turtle.fillcolor("white")
turtle.begin_fill()
turtle.circle(20)
turtle.end_fill()
# 画左眼珠
turtle.penup()
turtle.goto(-30, 130)
turtle.pendown()
turtle.fillcolor("black")
turtle.begin_fill()
turtle.circle(10)
turtle.end_fill()
# 画右眼珠
turtle.penup()
turtle.goto(50, 130)
turtle.pendown()
turtle.fillcolor("black")
turtle.begin_fill()
turtle.circle(10)
turtle.end_fill()
# 画鼻子
turtle.penup()
turtle.goto(0, 80)
turtle.pendown()
turtle.setheading(270)
turtle.fillcolor("black")
turtle.begin_fill()
turtle.circle(20, 180)
turtle.end_fill()
# 画嘴巴
turtle.penup()
turtle.goto(-60, 20)
turtle.pendown()
turtle.setheading(-27)
turtle.circle(70, 55)
# 完成
turtle.done()
```
python代码画帕恰狗
很好的问题!可以使用Python中的matplotlib库来实现。以下是一个例子:
```python
import matplotlib.pyplot as plt
import numpy as np
# 设置坐标轴范围
x = np.arange(-2, 2, 0.01)
y = np.arange(-2, 2, 0.01)
# 创建网格
X, Y = np.meshgrid(x, y)
# 定义函数
Z = X*np.exp(-X**2 - Y**2)
# 绘制图形
plt.contour(X, Y, Z, cmap='binary')
plt.axis('off')
plt.show()
```
这段代码将画出帕恰狗的轮廓。你也可以根据自己的喜好更改颜色和样式,添加标签等。希望能对你有所帮助!
相关推荐







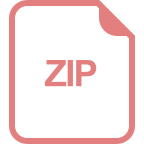
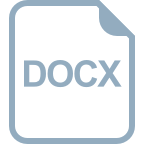