写一段python代码用numpy和pil将图片尺寸改为28*28像素
时间: 2024-09-11 07:05:53 浏览: 102
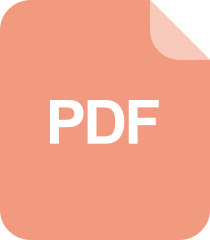
Python 使用PIL numpy 实现拼接图片的示例
当然可以。首先,你需要安装`numpy`和`Pillow`库(如果还没安装的话)。这里是一个简单的Python代码示例,用于使用`numpy`和`Pillow`改变图像尺寸:
```python
from PIL import Image
import numpy as np
# 打开图片文件
def resize_image(image_path):
# 使用Pillow打开图片
with Image.open(image_path) as im:
# 将图片转换为灰度图(如果需要)
if im.mode != 'L':
im = im.convert('L')
# 调整大小为28x28像素
resized_im = im.resize((28, 28))
# 将调整后的PIL图像转换为numpy数组
resized_array = np.array(resized_im)
return resized_array
# 使用函数处理图片
image_path = "path_to_your_image.jpg" # 替换为你的图片路径
resized_image = resize_image(image_path)
```
在这个例子中,我们先读取图片,然后通过`.resize()`方法将其大小更改为28x28像素,最后转换为numpy数组以便进一步处理。
阅读全文
相关推荐
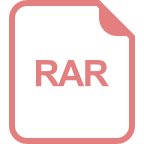
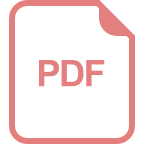















