用分支结构编写程序判断字符类型
时间: 2024-10-26 18:04:01 浏览: 6
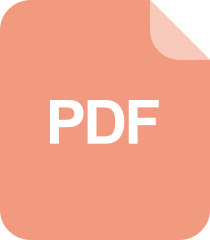
Java分支结构和循环结构原理与用法详解
在编程中,分支结构(如if-else、switch-case等)常用于根据输入的不同条件判断字符类型。下面是一个简单的Python示例,用于判断字符是否是字母、数字还是其他符号:
```python
def judge_char_type(char):
if isinstance(char, str): # 判断字符是否是字符串类型
if char.isalpha(): # 判断字符是否为字母
print(f"{char}是字母")
elif char.isdigit(): # 判断字符是否为数字
print(f"{char}是数字")
else: # 其他情况(包括空格、标点符号等)
print(f"{char}不是字母也不是数字,可能是其他符号")
# 测试
judge_char_type('A') # 输出: A是字母
judge_char_type('5') # 输出: 5是数字
judge_char_type('.') # 输出: .不是字母也不是数字,可能是其他符号
```
阅读全文
相关推荐
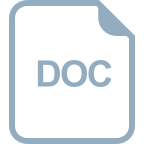
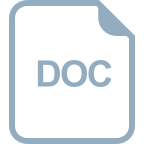















