html 如何将鼠标的指针替换为图片显示 完整示例代码
时间: 2024-09-13 17:18:15 浏览: 14
在HTML中,如果你想将鼠标指针替换为图片,你可以使用CSS的`cursor`属性,并指定一张图片的URL作为指针。这里是一个简单的示例代码,演示如何将鼠标指针替换为一张图片:
```html
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<title>自定义鼠标指针示例</title>
<style>
/* 定义一个类选择器,用来指定自定义指针 */
.custom-cursor {
cursor: url('cursor.png'), auto; /* 指定图片路径和备用指针类型 */
}
</style>
</head>
<body>
<div class="custom-cursor" style="width: 200px; height: 200px; background-color: #f0f0f0;">
鼠标悬停在此区域查看自定义指针效果
</div>
</body>
</html>
```
在这个例子中,`.custom-cursor` 类被用来应用自定义的鼠标指针效果。你需要将`cursor.png`替换为你自己的图片路径。`auto`是一个备用选项,它确保如果指定的图片无法加载,浏览器会使用默认的指针。
请注意,图片路径应该是正确的,并且图片文件应该可访问。此外,浏览器对自定义指针的支持可能有限制,比如图片尺寸和格式等。
相关问题
html鼠标指针悬停图片放大
以下是使用HTML和CSS实现鼠标指针悬停图片放大的示例:
```html
<!DOCTYPE html>
<html>
<head>
<style>
.container {
position: relative;
width: 200px;
height: 200px;
overflow: hidden;
}
.image {
display: block;
width: 100%;
height: auto;
transition: transform 0.3s ease;
}
.container:hover .image {
transform: scale(1.2);
}
</style>
</head>
<body>
<div class="container">
<img class="image" src="your-image.jpg" alt="Your Image">
</div>
</body>
</html>
```
在上面的示例中,我们创建了一个容器(`<div class="container">`),并在其中放置了一个图片(`<img class="image" src="your-image.jpg" alt="Your Image">`)。通过CSS的`transform`属性和`scale`函数,我们实现了鼠标悬停时图片的放大效果。当鼠标指针悬停在容器上时,图片会以1.2倍的比例进行放大。
请注意,你需要将`src`属性的值替换为你自己的图片路径。
html中使光标显示为段落上的指针的代码
在HTML中,可以使用CSS来改变鼠标指针的样式。要在段落上显示指针形状的光标,可以在CSS中使用以下代码:
```css
p {
cursor: pointer;
}
```
这将使所有段落元素的光标显示为指针形状。可以将`p`替换为其他元素选择器,以更改其他元素的光标形状。
相关推荐
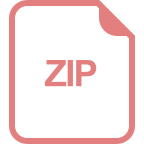
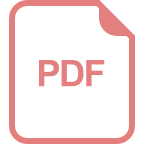
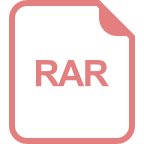












