小明老鼠放奶酪c语言
时间: 2024-12-04 20:12:40 浏览: 16
在C语言中,我们通常不会直接描述一个小明老鼠放奶酪的故事,因为这涉及到复杂的变量、函数以及面向对象的概念,而C语言是一种过程式编程语言,更适合编写控制流程和数据处理的程序。不过,如果要用简单的例子来说明如何在C语言中模拟这个场景,我们可以创建一个"动物"和"奶酪"的抽象模型。
```c
#include <stdio.h>
// 定义一个结构体表示奶酪
typedef struct {
int value;
} Cheese;
// 定义一个抽象的动物结构体,其中包含放奶酪的行为
typedef void (*Action)(Animal *, Cheese *);
// 定义鼠类,继承自动物,并实现放奶酪的方法
struct Mouse {
Animal base; // 基础动物类型
Action eatCheese;
};
// 动物基类的行为抽象
struct Animal {
Action action;
};
// 鼠类放奶酪的具体实现
void mouseEatCheese(Mouse *mouse, Cheese cheese) {
printf("小明老鼠正在吃一块价值%d的奶酪\n", cheese.value);
}
int main() {
Mouse mouse;
Cheese cheese = {50}; // 假设奶酪值为50
mouse.base.action = &mouseEatCheese; // 给动物赋予吃奶酪的行为
mouse.eatCheese(&mouse, cheese); // 调用老鼠的放奶酪方法
return 0;
}
```
在这个例子中,`Mouse` 类是一个子类,实现了 `Animal` 的 `eatCheese` 函数。当你实例化 `Mouse` 并给它分配吃奶酪的行为时,你可以像操作真正的 "小明老鼠" 一样让它放奶酪。
阅读全文
相关推荐
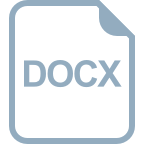
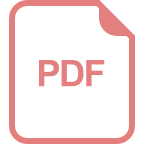
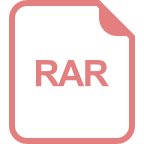
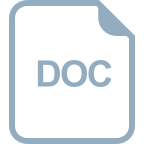


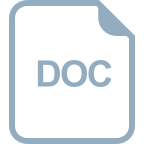
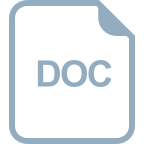
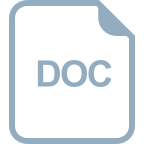
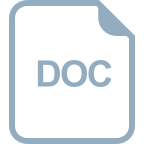
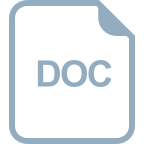







