pthread计算斐波那契数列
时间: 2024-12-04 18:26:12 浏览: 15
在使用POSIX线程(pthread)库来计算斐波那契数列时,可以创建一个单独的线程函数,该函数负责递归地计算斐波那契数,并通过共享内存或者其他同步机制将结果传递给主线程。下面是一个简单的示例,展示了如何使用pthread创建一个子线程来计算斐波那契数:
```c
#include <stdio.h>
#include <pthread.h>
// 全局变量用于存储斐波那契值
int fib_result;
// 计算斐波那契数的辅助函数
void* fibonacci_thread(void* arg) {
int n = *(int*)arg;
if (n <= 1)
return &fib_result;
else
return fib_result = *(int*)((fibonacci_thread((void*)(n - 1))) + fib_result);
}
void calculate_fib(int n) {
pthread_t thread_id;
pthread_create(&thread_id, NULL, fibonacci_thread, &n);
// 等待子线程完成并获取结果
pthread_join(thread_id, NULL);
printf("Fibonacci of %d is: %d\n", n, fib_result);
}
int main() {
int num = 10; // 需要计算的斐波那契数
calculate_fib(num);
return 0;
}
```
在这个例子中,`fibonacci_thread` 函数作为线程目标函数,`calculate_fib` 函数启动线程并等待它完成。注意,由于递归深度可能很深,如果n很大,这种方法可能会导致栈溢出。
阅读全文
相关推荐
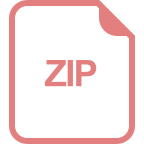
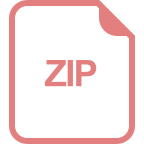
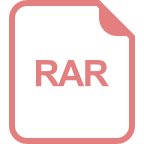

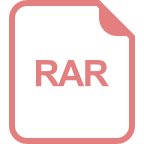
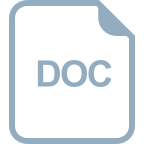



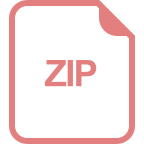
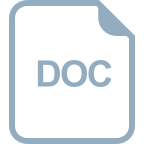
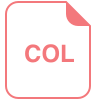


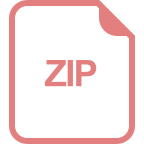