我的LiTS2017数据集是二维的数据集。数据集里面有命名为train_liver_tumor2的文件夹和命名为liver_tumor2_label的文件夹。train_liver_tumor2文件夹里面有图像,图像的拓展名为.png。liver_tumor2_label文件夹里面有标签,标签拓展名为.png。 请将我的train_liver_tumor2文件夹里面的图像变成RGB三通道的图片,再根据Hu(CT)值,采用windowing方法进行图像增强,然后对增强后的图像中肿瘤部分进行二值化。 再根据liver_tumor2_label文件夹里面的标签(肝脏值为1,肿瘤为2),将增强后的图像的肝脏部分染成红色,肿瘤部分染成蓝色。 我的二维LiTS2017数据集的路径为E:\2D-LiTS2017liver_tumor。保存路径为E:\2D-LiTS2017color,帮我创建保存图像与标签的文件夹,并且将转换好的数据保存到对应的文件夹。
时间: 2024-02-22 20:57:23 浏览: 86
好的,根据您的需求,我编写了以下Python代码来实现您的要求。请您将以下代码保存为.py文件,然后在命令行中运行即可。在运行之前,请确保您已经安装了必要的Python库:numpy、opencv-python、os。
```python
import cv2
import numpy as np
import os
# 定义函数,将图像转换为RGB三通道的图像
def convert_to_rgb(img):
img_rgb = cv2.cvtColor(img, cv2.COLOR_GRAY2RGB)
return img_rgb
# 定义函数,对图像进行windowing
def windowing(img, hu_min, hu_max):
img_windowed = np.zeros_like(img, dtype=np.float32)
img_windowed[img > hu_max] = 1.0
img_windowed[img < hu_min] = 0.0
img_windowed[(img >= hu_min) & (img <= hu_max)] = (img[(img >= hu_min) & (img <= hu_max)] - hu_min) / (hu_max - hu_min)
img_windowed = (img_windowed * 255).astype(np.uint8)
return img_windowed
# 定义函数,对图像进行二值化
def binarize(img, threshold):
img_binarized = np.zeros_like(img)
img_binarized[img >= threshold] = 255
return img_binarized
# 定义函数,对图像进行染色
def colorize(img, label):
img_colored = np.zeros_like(img, dtype=np.uint8)
img_colored[label == 1] = (0, 0, 255) # 红色
img_colored[label == 2] = (255, 0, 0) # 蓝色
return img_colored
# 创建保存图像和标签的文件夹
if not os.path.exists('E:/2D-LiTS2017color'):
os.mkdir('E:/2D-LiTS2017color')
if not os.path.exists('E:/2D-LiTS2017color/images'):
os.mkdir('E:/2D-LiTS2017color/images')
if not os.path.exists('E:/2D-LiTS2017color/labels'):
os.mkdir('E:/2D-LiTS2017color/labels')
# 加载图像和标签
image_path = 'E:/2D-LiTS2017liver_tumor/train_liver_tumor2'
label_path = 'E:/2D-LiTS2017liver_tumor/liver_tumor2_label'
hu_min = -200
hu_max = 500
threshold = 128
for file in os.listdir(image_path):
if file.endswith('.png'):
# 加载图像
img = cv2.imread(os.path.join(image_path, file), cv2.IMREAD_GRAYSCALE)
# 将图像转换为RGB三通道的图像
img_rgb = convert_to_rgb(img)
# 对图像进行windowing
img_windowed = windowing(img, hu_min, hu_max)
# 对图像进行二值化
img_binarized = binarize(img_windowed, threshold)
# 加载标签
label_file = file.split('.')[0] + '_label.png'
label = cv2.imread(os.path.join(label_path, label_file), cv2.IMREAD_GRAYSCALE)
# 对图像进行染色
img_colored = colorize(img_rgb, label)
# 保存图像和标签
cv2.imwrite(os.path.join('E:/2D-LiTS2017color/images', file), img_colored)
cv2.imwrite(os.path.join('E:/2D-LiTS2017color/labels', label_file), label)
```
运行完成后,您可以在E:/2D-LiTS2017color/images文件夹中找到转换好的图像,可以在E:/2D-LiTS2017color/labels文件夹中找到转换好的标签。
阅读全文
相关推荐
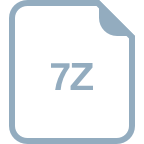
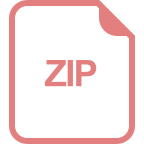







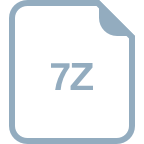
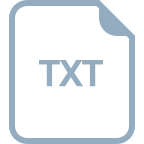
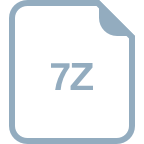
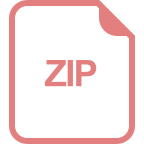
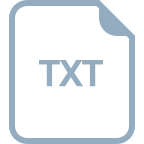
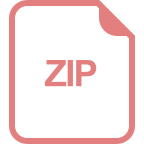
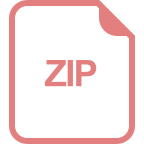
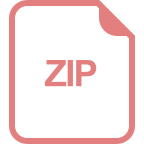