用 c++写一个 helloworld带注释
时间: 2023-04-08 18:02:57 浏览: 136
//这是一个简单的C程序,输出Hello World!
#include <stdio.h> //包含标准输入输出头文件
int main() { //程序入口
printf("Hello World!\n"); //输出Hello World!
return 0; //返回0表示程序正常结束
}
相关问题
c++编写程序输出一行文字:hello,world。 注释:“hello,world”程序是指在计算机屏幕上输出“hello,world”这行字符串的计算机程序。
要写一个C程序输出一行文字"hello,world",可以使用以下代码之一:
1. 使用iostream头文件和命名空间std:
#include <iostream>
using namespace std;
int main() {
cout << "hello,world" << endl;
return 0;
}
2. 使用stdio.h头文件:
#include <stdio.h>
int main() {
printf("hello,world\n");
return 0;
}
这两个代码片段都可以实现在计算机屏幕上输出"hello,world"这行字符串的功能。选择哪个代码片段取决于你的编程环境和个人偏好。
使用c++写一个OLED显示驱动程序加上注释
非常感谢您的问题,我可以回答这个问题。以下是一个使用 C 语言编写的 OLED 显示驱动程序的示例代码,其中包括注释:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define OLED_I2C_ADDR x3C // OLED 显示器的 I2C 地址
// OLED 显示器的初始化命令序列
const unsigned char init_cmds[] = {
xAE, // 关闭显示
xD5, x80, // 设置时钟分频因子,震荡器频率
xA8, x3F, // 设置驱动路数
xD3, x00, // 设置显示偏移
x40, // 设置显示开始行 [5:]
x8D, x14, // 启用电荷泵
x20, x00, // 设置内存地址模式
xA1, // 指定段重定向
xC8, // 设置扫描方向
xDA, x12, // 设置 COM 硬件引脚配置
x81, xCF, // 设置对比度
xD9, xF1, // 设置预充电周期
xDB, x40, // 设置 VCOMH 电压倍率
xA4, // 关闭全局显示
xA6, // 设置显示方式
xAF // 打开显示
};
// 初始化 OLED 显示器
void oled_init(int fd) {
int i;
for (i = ; i < sizeof(init_cmds); i++) {
unsigned char cmd[2] = {x00, init_cmds[i]};
if (write(fd, cmd, sizeof(cmd)) != sizeof(cmd)) {
perror("write");
exit(1);
}
}
}
// 在 OLED 显示器上显示一行文本
void oled_write_line(int fd, int line, const char *text) {
int i;
unsigned char cmd[2] = {x00, xB + line};
if (write(fd, cmd, sizeof(cmd)) != sizeof(cmd)) {
perror("write");
exit(1);
}
cmd[] = x40;
for (i = ; i < 16; i++) {
if (*text) {
cmd[1] = *text++;
} else {
cmd[1] = ' ';
}
if (write(fd, cmd, sizeof(cmd)) != sizeof(cmd)) {
perror("write");
exit(1);
}
}
}
int main(int argc, char *argv[]) {
int fd;
const char *dev = "/dev/i2c-1";
if ((fd = open(dev, O_RDWR)) < ) {
perror("open");
exit(1);
}
if (ioctl(fd, I2C_SLAVE, OLED_I2C_ADDR) < ) {
perror("ioctl");
exit(1);
}
oled_init(fd);
oled_write_line(fd, , "Hello, world!");
close(fd);
return ;
}
```
希望这个示例代码能够对您有所帮助。
阅读全文
相关推荐
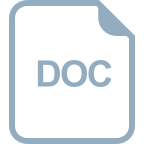
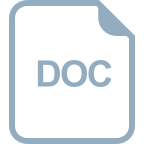
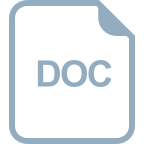
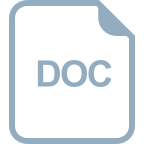
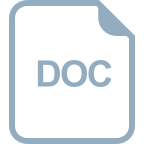
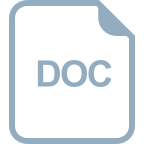
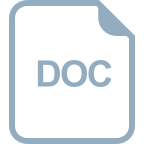
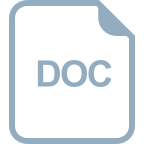
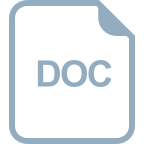
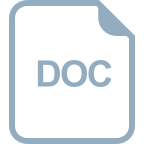
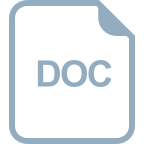
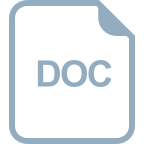
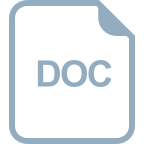
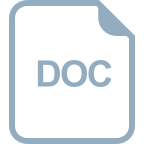