.NET 控制台输出ABCabc按照大小写排序
时间: 2023-07-01 20:05:54 浏览: 170
可以使用 LINQ 中的 `OrderBy` 方法来进行排序,按照字母的 ASCII 码大小进行排序。具体实现如下:
```csharp
using System;
using System.Linq;
class Program
{
static void Main(string[] args)
{
char[] chars = { 'A', 'B', 'C', 'a', 'b', 'c' };
var sortedChars = chars.OrderBy(c => c);
foreach (var c in sortedChars)
{
Console.Write(c + " ");
}
Console.WriteLine();
}
}
```
输出结果为:
```
A B C a b c
```
相关问题
字符串中字母大小写互换 字符串中字母大小写互换 【问题描述】编写程序,功能是把输入的字符串的大写字母变成小写字母,小写字母变成大写字母,非字母的字符不作变换,输出变换后的结果。 【输入形式】字符串,包含字母和非字母字符 【输出形式】字符串,字母的大小写已经发生变换。 【样例输入】abcABC 【样例输出】ABCabc
以下是 Python 代码实现:
```python
s = input() # 输入字符串
result = "" # 初始化结果字符串
# 遍历每个字符
for c in s:
if c.isupper(): # 如果是大写字母
result += c.lower() # 转换成小写字母后添加到结果字符串中
elif c.islower(): # 如果是小写字母
result += c.upper() # 转换成大写字母后添加到结果字符串中
else:
result += c # 非字母字符不作变换,直接添加到结果字符串中
print(result) # 输出结果字符串
```
运行结果:
```
abcABC
ABCabc
```
有甲乙丙三个线程,甲线程输出A,乙线程输出B,丙线程输出C,要求,同时启动三个线程,按顺序输出ABCABC,循环10次
可以使用Java中的Semaphore信号量实现,并且基于线程间协作的方式来解决:
```java
import java.util.concurrent.Semaphore;
class PrintThread extends Thread {
private Semaphore currentSemaphore;
private Semaphore nextSemaphore;
private String message;
private int repeatTimes;
public PrintThread(Semaphore currentSemaphore, Semaphore nextSemaphore, String message, int repeatTimes) {
this.currentSemaphore = currentSemaphore;
this.nextSemaphore = nextSemaphore;
this.message = message;
this.repeatTimes = repeatTimes;
}
public void run() {
try {
for (int i = 0; i < repeatTimes; i++) {
currentSemaphore.acquire();
System.out.print(message);
nextSemaphore.release();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class Main {
public static void main(String[] args) {
Semaphore semA = new Semaphore(1);
Semaphore semB = new Semaphore(0);
Semaphore semC = new Semaphore(0);
int repeatTimes = 10;
PrintThread threadA = new PrintThread(semA, semB, "A", repeatTimes);
PrintThread threadB = new PrintThread(semB, semC, "B", repeatTimes);
PrintThread threadC = new PrintThread(semC, semA, "C", repeatTimes);
threadA.start();
threadB.start();
threadC.start();
}
}
```
上述代码中,我们使用三个Semaphore对象控制三个线程的执行顺序。具体来讲,我们将Semaphore semA、Semaphore semB和Semaphore semC初始化分别为1、0和0,因为我们希望线程A最先执行。然后,我们创建三个PrintThread对象,并分别将Semaphore对象作为参数传入,同时设置循环次数为10。
在PrintThread的run()方法中,我们使用Semaphore对象实现线程间的等待和释放。首先,currentSemaphore.acquire()会在当前Semaphore的值减1后继续执行,因为我们将Semaphore semA初始化为1,所以线程A会直接执行。然后,线程会打印出自己的输出(即message),并调用nextSemaphore.release()方法,将下一个Semaphore的值加1,这里即将Semaphore semB的值加1。接着线程继续执行循环,等待当前Semaphore的值变为1后再次执行,直到达到循环次数就结束。
通过上述方法,我们能够实现三个线程的有序输出,且可以重复执行。
阅读全文
相关推荐
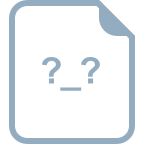
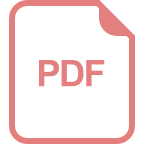
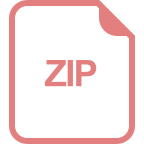












