re.search
时间: 2023-08-07 10:05:47 浏览: 99
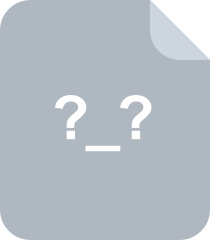
rejson.so redisearch.so
`re.search()` 是 Python 中用于在字符串中搜索匹配的正则表达式的函数。它返回一个匹配对象,如果找到了匹配项,则可以使用该对象的方法和属性来访问匹配项的信息。如果没有找到匹配项,它将返回 None。例如,下面的代码使用 `re.search()` 来查找字符串中是否包含数字:
```python
import re
string = "Hello, 123 World!"
match = re.search(r'\d+', string)
if match:
print("Found a match:", match.group())
else:
print("No match found.")
```
输出:
```
Found a match: 123
```
在这个例子中,`r'\d+'` 是一个正则表达式,它匹配一个或多个数字。`re.search()` 在 `string` 字符串中搜索这个正则表达式,并返回一个匹配对象。我们检查匹配对象是否存在,如果存在,我们使用 `match.group()` 方法来获取匹配项的值,即数字 `"123"`。
阅读全文
相关推荐
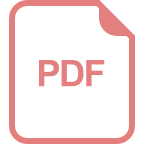
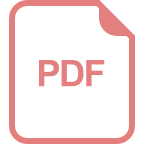
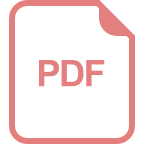
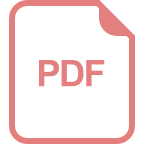













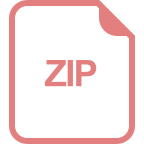