b) Make the clock show the current time. For this you should use the Calendar class from java.util with: ● Calendar.getInstance() - create Calendar object for current date/time ● get(field) - return integer field from Calendar object, where field may be Calendar.HOUR, Calendar.MINUTE, Calendar.SECOND, Calendar.AM_PM, etc. ● the value returned from Calendar.AM_PM may be compared with the values Calendar.AM and Calendar.PM to determine if it is before or after mid-day Create a function that runs continuously and updates the display to always show the current time.应该怎么写?
时间: 2024-02-05 19:03:27 浏览: 20
你可以使用以下代码实现一个实时更新的时钟:
```java
import java.util.Calendar;
public class Clock {
public static void main(String[] args) {
while (true) {
Calendar calendar = Calendar.getInstance();
int hour = calendar.get(Calendar.HOUR);
int minute = calendar.get(Calendar.MINUTE);
int second = calendar.get(Calendar.SECOND);
int amPm = calendar.get(Calendar.AM_PM);
String amPmStr = amPm == Calendar.AM ? "AM" : "PM";
String time = String.format("%02d:%02d:%02d %s", hour, minute, second, amPmStr);
System.out.print("\r" + time);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
在这个程序中,我们使用 `Calendar.getInstance()` 获取当前日期和时间的 `Calendar` 对象,并使用 `get()` 方法获取小时、分钟、秒和上午/下午的值。然后,我们使用 `String.format()` 方法格式化字符串,并使用 `System.out.print()` 方法在控制台上输出当前时间。通过使用 `\r`,我们可以在每秒更新时覆盖前一个时间的输出,从而创建实时更新的时钟。最后,我们使用 `Thread.sleep()` 方法暂停程序一秒钟,以便下一秒钟的时间可以被计算和输出。
相关推荐
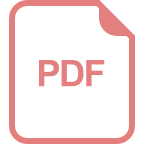
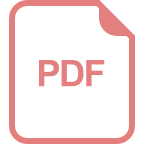















