对weatherdata.csv里的数据进行筛选,选出自己所在省份(直辖市)的数据,完成以下要求: 计算其每个地区当天的平均温度、湿度,在一张柱状图上表示出来 筛选出每个地区当天的(最高位温、最高湿度)和(最低温,
时间: 2024-09-19 22:03:53 浏览: 99
首先,为了对`weatherdata.csv`文件中的数据进行筛选并完成所提的要求,我们需要按照以下步骤操作:
1. **数据加载和预处理**:
使用pandas库读取CSV文件,假设文件包含`province`(省份)、`temperature`(温度)、`humidity`(湿度)等列。检查数据是否已清洗,确保`province`列包含了所有直辖市的信息。
```python
import pandas as pd
# 加载数据
df_weather = pd.read_csv('weatherdata.csv')
# 检查数据集中是否有直辖市信息
province_unique = df_weather['province'].unique()
if not '直辖市' in province_unique:
print("数据集中缺少直辖市的信息,请确认数据完整性。")
```
2. **筛选数据**:
创建一个新的DataFrame,只包含直辖市的数据。
```python
# 省份列表,这里假设直辖市有四个:北京、上海、天津和重庆
city_list = ['北京', '上海', '天津', '重庆']
df_city = df_weather[df_weather['province'].isin(city_list)]
```
3. **计算统计数据**:
分别计算每个地区每天的平均温度和湿度,以及最高和最低值。
```python
# 计算平均温度和湿度
average_temp = df_city.groupby(['province', 'date'])['temperature'].mean().reset_index()
average_humidity = df_city.groupby(['province', 'date'])['humidity'].mean().reset_index()
# 计算最高和最低值
max_min_data = df_city.groupby(['province', 'date']).agg({'temperature': {'max': 'max', 'min': 'min'},
'humidity': {'max': 'max', 'min': 'min'}}).reset_index()
```
4. **绘制柱状图**:
使用matplotlib或seaborn库创建柱状图,展示平均温度和湿度。
```python
import matplotlib.pyplot as plt
# 平均温度柱状图
plt.figure(figsize=(10,6))
for city, group in average_temp.groupby('province'):
plt.plot(group['date'], group['temperature'], label=city)
plt.xlabel('日期')
plt.ylabel('平均温度')
plt.title('各直辖市平均气温变化')
plt.legend()
plt.show()
# 同理,绘制湿度柱状图
plt.figure(figsize=(10,6))
for city, group in average_humidity.groupby('province'):
plt.plot(group['date'], group['humidity'], label=city)
plt.xlabel('日期')
plt.ylabel('平均湿度')
plt.title('各直辖市平均湿度变化')
plt.legend()
plt.show()
```
5. **合并数据并绘制综合图表**:
可能需要将上述两个结果合并在一起,以便在同一图表中显示最高和最低温度及湿度。
以上代码示例展示了基本流程,实际操作可能会因数据结构和缺失值处理有所不同。
阅读全文
相关推荐
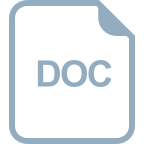
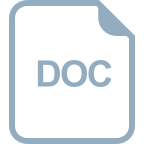
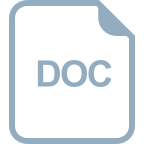



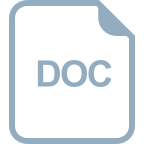
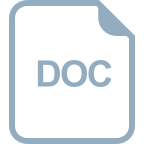
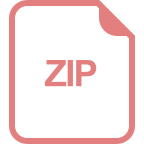