C#string放大10000倍再转float
时间: 2024-09-27 21:15:42 浏览: 40
在C#中,如果你想将一个字符串转换为浮点数,并将其放大10000倍,你需要首先确保字符串能成功解析为数字,然后乘以10000后再进行转换。如果字符串不是有效的数字格式,将会抛出异常。下面是一个简单的示例:
```csharp
string inputString = "123.45"; // 假设这是一个字符串
double number;
try {
number = double.Parse(inputString); // 将字符串转换为double类型
double amplifiedNumber = number * 10000; // 放大10000倍
float finalFloat = (float)amplifiedNumber; // 转换为float类型
Console.WriteLine($"放大10000倍后的浮点数: {finalFloat}");
} catch (FormatException) {
Console.WriteLine($"无法将'{inputString}'转换为数字.");
}
```
这段代码会尝试将输入字符串转换为double,如果转换失败,则捕获`FormatException`并输出错误消息。
相关问题
C#图形图像处理放大镜效果
### C# 图形图像处理实现放大镜效果
为了实现在C#应用程序中创建一个具有放大镜功能的效果,可以利用`SnsPictrueBoxSample`组件来简化操作。此组件不仅提供简易集成和高性能引擎的支持[^1],还允许开发者通过少量代码快速构建复杂的功能。
下面是一个完整的示例程序,展示了如何使用`SnsPictrueBoxSample`以及基本的Windows Forms技术,在鼠标悬停位置动态展示放大部分的内容:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
public class Magnifier : Form {
private PictureBox originalPictureBox; // 原始图片框
private Panel magnifiedPanel; // 显示放大的区域面板
private Timer timer; // 定时器用于更新放大视图
public Magnifier() {
InitializeComponents();
// 加载初始图片
string imagePath = "your_image_path.jpg";
Bitmap bitmap = new Bitmap(imagePath);
originalPictureBox.Image = bitmap;
// 设置定时器间隔为20ms(约每秒50次刷新),以保持平滑移动体验
timer.Interval = 20;
timer.Tick += UpdateMagnification;
timer.Start();
}
private void InitializeComponents() {
SuspendLayout();
// 初始化原始图片框
originalPictureBox = new SnsPictrueBoxSample(); // 使用自定义控件替代默认PictureBox
originalPictureBox.Dock = DockStyle.Fill;
Controls.Add(originalPictureBox);
// 创建透明背景的小窗口作为放大镜面罩
magnifiedPanel = new Panel {
Size = new Size(200, 200),
BackColor = Color.White,
BorderStyle = BorderStyle.FixedSingle,
Location = PointToClient(MousePosition), // 初始定位到屏幕中心
Visible = false // 默认隐藏直到需要显示时才激活
};
Controls.Add(magnifiedPanel);
// 添加事件监听以便于跟踪鼠标的动作
MouseMove += HandleMouseMoveEvent;
Deactivate += HideMagnifyingGlassOnDeactivation;
ResumeLayout(false);
}
/// <summary>
/// 当表单失去焦点时自动收起放大镜界面
/// </summary>
private void HideMagnifyingGlassOnDeactivation(object sender, EventArgs e) => magnifiedPanel.Visible = false;
/// <summary>
/// 处理鼠标移动事件并调整放大镜的位置及内容
/// </summary>
private void HandleMouseMoveEvent(object sender, MouseEventArgs e) {
if (!magnifiedPanel.Visible && !originalPictureBox.ClientRectangle.Contains(e.Location))
return;
var zoomFactor = 3f; // 放大倍数因子
Rectangle sourceRect = GetSourceRectangleForZooming(zoomFactor, e.X, e.Y);
using (Bitmap croppedBmp = CropImage((Bitmap)originalPictureBox.Image, sourceRect)) {
DrawScaledImage(croppedBmp, magnifiedPanel.CreateGraphics(), zoomFactor);
}
PositionMagnifierAtMouseLocation(e.Location);
ShowOrHideMagnifierBasedOnBounds(sourceRect);
}
/// <summary>
/// 获取要截取的源矩形范围
/// </summary>
private static Rectangle GetSourceRectangleForZooming(float factor, int mouseX, int mouseY) =>
new Rectangle(mouseX / factor - 50, mouseY / factor - 50, 100, 100);
/// <summary>
/// 裁剪指定大小的子图像
/// </summary>
private static Bitmap CropImage(Bitmap bmp, Rectangle rect) {
try {
return bmp.Clone(rect, bmp.PixelFormat);
} catch (Exception ex) when ((ex is ArgumentException || ex is IndexOutOfRangeException)) {
throw new InvalidOperationException("裁剪超出边界");
}
}
/// <summary>
/// 将缩小版位图画入目标绘图表面,并按比例缩放回原尺寸
/// </summary>
private static void DrawScaledImage(Image img, Graphics g, float scale) {
g.Clear(Color.White);
g.DrawImage(img, new Rectangle(Point.Empty, new Size(
Convert.ToInt32(img.Width * scale),
Convert.ToInt32(img.Height * scale))));
}
/// <summary>
/// 移动放大镜至当前光标所在处
/// </summary>
private void PositionMagnifierAtMouseLocation(Point mousePos) =>
magnifiedPanel.Location = new Point(mousePos.X + 20, mousePos.Y + 20);
/// <summary>
/// 根据是否越界决定是否显示或隐藏放大镜
/// </summary>
private void ShowOrHideMagnifierBasedOnBounds(Rectangle bounds) =>
magnifiedPanel.Visible = !(bounds.Left < 0 ||
bounds.Top < 0 ||
bounds.Right >= originalPictureBox.ClientSize.Width ||
bounds.Bottom >= originalPictureBox.ClientSize.Height);
/// <summary>
/// 更新放大视图的方法由计时器触发执行
/// </summary>
private void UpdateMagnification(object sender, EventArgs e) {
Cursor.Position = PointToScreen(Cursor.Position); // 强制刷新游标坐标
Invalidate(true); // 请求重绘整个窗体
}
}
```
这段代码实现了当用户将鼠标指针放在图片上的时候,会弹出一个小窗口显示出该点周围被放大的部分;随着鼠标的移动,这个小窗口也会随之改变其内部所呈现的画面片段。这正是典型的放大镜行为模拟。
阅读全文
相关推荐



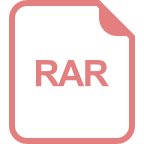

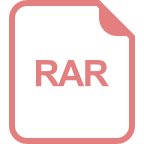











