springboot 本地文件上传下载
时间: 2023-03-30 14:00:23 浏览: 101
Spring Boot 提供了很多方便的工具来实现本地文件上传和下载。你可以使用 Spring Boot 的 MultipartFile 类来处理上传的文件,使用 Resource 类来处理下载的文件。具体实现可以参考 Spring Boot 官方文档或者相关的教程。
相关问题
springboot本地文件上传后获取文件路径
在Spring Boot应用中,处理文件上传通常涉及前端将文件发送到服务器,然后后端保存文件并返回文件路径给客户端。以下是简单的步骤:
1. 配置MultipartFile:首先,在Controller层的请求处理器上,你需要声明一个`@RequestParam MultipartFile file`,这是Spring MVC用来接收文件上传的对象。
```java
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
// 文件验证和处理逻辑...
}
```
2. 保存文件:Spring提供了一些内置的方式保存文件,如使用`TemporaryUploadedFile`临时存储,或者直接写入到指定目录。例如,你可以创建一个临时文件目录,然后调用`save()`方法:
```java
@Autowired
private FileSystemResource resource;
try {
String filename = file.getOriginalFilename();
Path filePath = Paths.get("uploads/" + filename);
resource.copy(file.getInputStream(), new FileOutputStream(filePath.toFile()));
return "success" + filePath.toUri(); // 返回文件URI,即路径
} catch (IOException e) {
throw new RuntimeException(e);
}
```
这里假设有一个名为"uploads"的目录用于存放上传文件。
springboot实现文件上传下载步骤
好的,我可以帮您回答关于Spring Boot实现文件上传下载的步骤。
1. 依赖引入
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-io</artifactId>
<version>1.3.2</version>
</dependency>
```
2. 配置文件
在application.properties文件中添加以下配置:
```
spring.servlet.multipart.max-file-size=128MB
spring.servlet.multipart.max-request-size=256MB
```
3. 文件上传
在Controller中添加以下代码:
```
@PostMapping("/uploadFile")
public String uploadFile(@RequestParam("file") MultipartFile file, RedirectAttributes redirectAttributes) {
String fileName = StringUtils.cleanPath(file.getOriginalFilename());
try {
// 保存文件到本地
FileCopyUtils.copy(file.getInputStream(), new FileOutputStream("upload/" + fileName));
} catch (IOException e) {
e.printStackTrace();
}
redirectAttributes.addFlashAttribute("message", "You successfully uploaded " + fileName + "!");
return "redirect:/uploadStatus";
}
```
4. 文件下载
在Controller中添加以下代码:
```
@GetMapping("/downloadFile/{fileName:.+}")
public ResponseEntity<Resource> downloadFile(@PathVariable String fileName, HttpServletRequest request) {
// 加载文件资源
Resource resource = loadFileAsResource(fileName);
// 确定MIME类型
String contentType = null;
try {
contentType = request.getServletContext().getMimeType(resource.getFile().getAbsolutePath());
} catch (IOException ex) {
ex.printStackTrace();
}
// 默认MIME类型为二进制流
if (contentType == null) {
contentType = "application/octet-stream";
}
// 构建响应
return ResponseEntity.ok()
.contentType(MediaType.parseMediaType(contentType))
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
}
private Resource loadFileAsResource(String fileName) {
Resource resource = new FileSystemResource("upload/" + fileName);
if (resource.exists()) {
return resource;
} else {
throw new RuntimeException("File not found: " + fileName);
}
}
```
以上就是Spring Boot实现文件上传下载的步骤。如果您有任何疑问或需求,请随时告诉我。
阅读全文
相关推荐
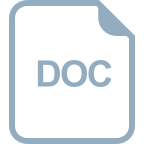
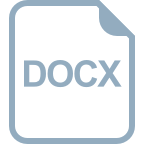
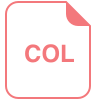
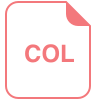

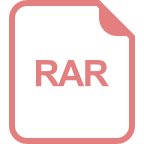
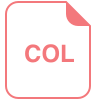




