pyside2 视频流 外加直方图
时间: 2023-10-24 18:08:47 浏览: 91
好的,如果您想在Pyside2应用程序中添加视频流并显示带刻度的直方图,您可以使用OpenCV库来捕获和处理视频流,并使用Matplotlib库来绘制直方图。
下面是一个简单的例子,可以帮助您开始:
```python
import sys
import cv2
from matplotlib.backends.backend_qt5agg import FigureCanvasQTAgg as FigureCanvas
from matplotlib.figure import Figure
from PySide2.QtWidgets import QApplication, QWidget, QVBoxLayout, QLabel
class VideoPlayer(QWidget):
def __init__(self, video_source=0):
super().__init__()
# Open the video source
self.video = cv2.VideoCapture(video_source)
# Create a figure for the histogram
self.figure = Figure()
self.canvas = FigureCanvas(self.figure)
self.ax = self.figure.add_subplot(111)
# Create a label for displaying the video frames
self.image_label = QLabel(self)
# Set up the layout
layout = QVBoxLayout()
layout.addWidget(self.image_label)
layout.addWidget(self.canvas)
self.setLayout(layout)
# Start the timer to update the video frames and histogram
self.timer = QTimer()
self.timer.timeout.connect(self.update_frame)
self.timer.start(30)
def update_frame(self):
# Read the next frame from the video stream
ret, frame = self.video.read()
if ret:
# Convert the frame to grayscale and calculate the histogram
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
hist, bins = np.histogram(gray.ravel(), 256, [0, 256])
# Update the histogram plot
self.ax.clear()
self.ax.bar(bins[:-1], hist, width=1)
self.ax.set_xlim([0, 256])
self.ax.set_ylim([0, 5000])
self.canvas.draw()
# Convert the frame to QImage format and display it
height, width, channels = frame.shape
bytes_per_line = channels * width
qimg = QImage(frame.data, width, height, bytes_per_line, QImage.Format_BGR888)
pixmap = QPixmap.fromImage(qimg)
self.image_label.setPixmap(pixmap)
if __name__ == '__main__':
app = QApplication(sys.argv)
player = VideoPlayer()
player.show()
sys.exit(app.exec_())
```
在这个例子中,我们创建了一个名为`VideoPlayer`的自定义QWidget类,它包含一个用于显示视频帧的QLabel和一个用于显示直方图的Matplotlib图表。我们使用OpenCV库来捕获和处理视频流,并使用Matplotlib库来计算和绘制直方图。我们还使用PySide2的QTimer类来定期更新视频帧和直方图。
请注意,这只是一个简单的例子,您需要根据您的应用程序需求进行修改。
阅读全文
相关推荐
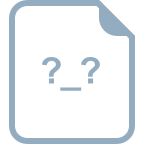
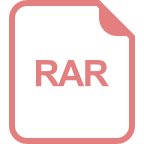
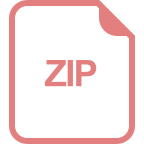
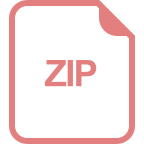
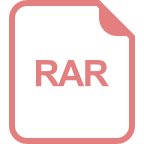
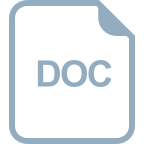
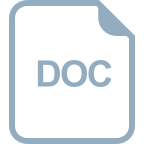











