不使用sklearn库实现以上问题
时间: 2024-05-12 16:20:33 浏览: 52
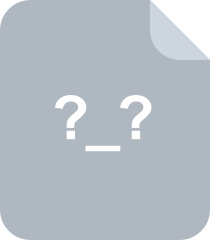
DBSCAN算法实践(基于Python实现,非调用sklearn库)
以下是不使用sklearn库的解决方案:
1. 逻辑回归:
首先,定义sigmoid函数:
```python
def sigmoid(z):
return 1 / (1 + np.exp(-z))
```
接着,定义代价函数和梯度下降算法:
```python
def cost_function(theta, X, y):
m = len(y)
h = sigmoid(X @ theta)
cost = (1 / m) * np.sum(-y * np.log(h) - (1 - y) * np.log(1 - h))
grad = (1 / m) * (X.T @ (h - y))
return cost, grad
def gradient_descent(X, y, theta, alpha, num_iters):
m = len(y)
J_history = []
for i in range(num_iters):
cost, grad = cost_function(theta, X, y)
theta = theta - (alpha * grad)
J_history.append(cost)
return theta, J_history
```
最后,将数据集拆分为训练集和测试集,然后执行以下代码:
```python
# 构建特征矩阵和标签向量
X = np.column_stack((np.ones(m), data[:, :-1]))
y = data[:, -1]
# 初始化参数
initial_theta = np.zeros(X.shape[1])
# 训练模型
theta, J_history = gradient_descent(X_train, y_train, initial_theta, 0.01, 1000)
# 预测测试集
y_pred = sigmoid(X_test @ theta)
# 计算准确率
accuracy = np.mean((y_pred >= 0.5) == y_test)
print(f"Accuracy: {accuracy}")
```
2. 线性回归:
首先,定义代价函数和梯度下降算法:
```python
def cost_function(theta, X, y):
m = len(y)
h = X @ theta
cost = (1 / (2 * m)) * np.sum((h - y) ** 2)
grad = (1 / m) * (X.T @ (h - y))
return cost, grad
def gradient_descent(X, y, theta, alpha, num_iters):
m = len(y)
J_history = []
for i in range(num_iters):
cost, grad = cost_function(theta, X, y)
theta = theta - (alpha * grad)
J_history.append(cost)
return theta, J_history
```
最后,将数据集拆分为训练集和测试集,然后执行以下代码:
```python
# 构建特征矩阵和标签向量
X = np.column_stack((np.ones(m), data[:, :-1]))
y = data[:, -1]
# 初始化参数
initial_theta = np.zeros(X.shape[1])
# 训练模型
theta, J_history = gradient_descent(X_train, y_train, initial_theta, 0.01, 1000)
# 预测测试集
y_pred = X_test @ theta
# 计算均方误差
mse = np.mean((y_pred - y_test) ** 2)
print(f"MSE: {mse}")
```
阅读全文
相关推荐
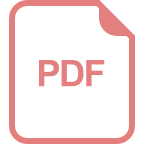
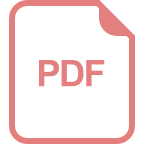
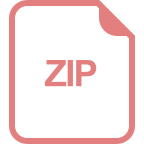
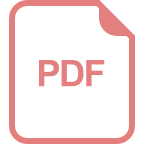
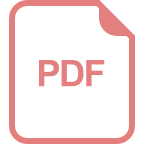
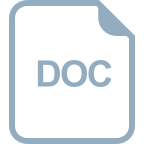
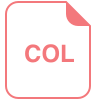








