将txt转为csv
时间: 2023-08-30 16:06:44 浏览: 46
可以使用Python的pandas库将txt文件转换为csv文件。
以下是一个示例代码:
```python
import pandas as pd
# 读取txt文件
df = pd.read_csv('data.txt', sep='\t', header=None)
# 将数据保存为csv文件
df.to_csv('data.csv', index=False)
```
在这个示例中,我们首先使用`read_csv()`方法读取txt文件,其中的`sep`参数指定了txt文件中的分隔符,`header=None`表示txt文件没有列名。然后使用`to_csv()`方法将数据保存为csv文件,其中`index=False`表示不保存行索引。
相关问题
python批量将txt转为csv
好的,可以使用Python中的pandas库来实现批量将txt文件转为csv文件的操作。具体步骤可以参考以下代码:
```python
import os
import pandas as pd
# 设置源文件目录和目标文件目录
source_dir = "./source/"
target_dir = "./target/"
# 遍历源文件目录中的所有txt文件,逐个进行转换
for filename in os.listdir(source_dir):
if filename.endswith(".txt"):
# 读取txt文件并转为DataFrame格式
file_path = os.path.join(source_dir, filename)
df = pd.read_csv(file_path, sep="\t")
# 生成目标文件路径并保存为csv格式
target_path = os.path.join(target_dir, filename.replace(".txt", ".csv"))
df.to_csv(target_path, index=False)
```
代码中首先设置了源文件目录和目标文件目录,然后使用os库的listdir()函数遍历源文件目录中的所有txt文件。对于每一个txt文件,我们使用pandas库的read_csv()函数读取为DataFrame格式,并将其保存为csv格式,最终存储到目标文件目录中。
以上是批量将txt转为csv的Python代码,希望能对您有所帮助。
用python将txt文本转为csv
可以使用Python中的pandas库来将txt文本转为csv格式。具体步骤如下:
1. 导入pandas库
```python
import pandas as pd
```
2. 读取txt文件
```python
df = pd.read_csv('filename.txt', sep='\t', header=None)
```
其中,filename.txt为要转换的txt文件名,sep='\t'表示txt文件中的分隔符为制表符,header=None表示txt文件中没有列名。
3. 将数据保存为csv文件
```python
df.to_csv('filename.csv', index=False)
```
其中,filename.csv为要保存的csv文件名,index=False表示不保存行索引。
通过以上步骤,就可以将txt文本转为csv格式了。
相关推荐
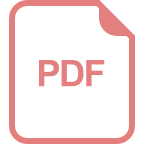
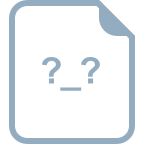
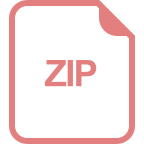












