简易计算器c++语言可计算小数利用栈
时间: 2023-07-16 18:11:26 浏览: 75
好的,以下是一个简易的计算器 C++ 代码,可计算小数,并使用栈实现:
```c++
#include<iostream>
#include<stack>
#include<string>
#include<cmath>
using namespace std;
bool isOperator(char c);
double calculate(double a, double b, char op);
int main() {
stack<double> numStack;
stack<char> opStack;
string str;
cin >> str;
int len = str.length();
double num = 0.0;
bool decimal = false;
int decimalCount = 0;
for (int i = 0; i < len; i++) {
char c = str[i];
if (isdigit(c)) {
if (decimal) {
num += (c - '0') * pow(10, -decimalCount);
decimalCount++;
}
else {
num = num * 10 + (c - '0');
}
}
else if (c == '.') {
decimal = true;
}
else if (isOperator(c)) {
numStack.push(num);
num = 0.0;
decimal = false;
decimalCount = 0;
while (!opStack.empty() && opStack.top() != '(' && ((c != '^' && isOperator(opStack.top())) || (c == '^' && opStack.top() == '^'))) {
char op = opStack.top();
opStack.pop();
double b = numStack.top();
numStack.pop();
double a = numStack.top();
numStack.pop();
numStack.push(calculate(a, b, op));
}
opStack.push(c);
}
else if (c == '(') {
opStack.push(c);
}
else if (c == ')') {
numStack.push(num);
num = 0.0;
decimal = false;
decimalCount = 0;
while (opStack.top() != '(') {
char op = opStack.top();
opStack.pop();
double b = numStack.top();
numStack.pop();
double a = numStack.top();
numStack.pop();
numStack.push(calculate(a, b, op));
}
opStack.pop();
}
}
numStack.push(num);
while (!opStack.empty()) {
char op = opStack.top();
opStack.pop();
double b = numStack.top();
numStack.pop();
double a = numStack.top();
numStack.pop();
numStack.push(calculate(a, b, op));
}
cout << numStack.top() << endl;
return 0;
}
bool isOperator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/' || c == '^';
}
double calculate(double a, double b, char op) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
return a / b;
case '^':
return pow(a, b);
default:
return 0.0;
}
}
```
代码中首先定义了一个数字栈 `numStack` 和一个操作符栈 `opStack`,然后读入一个字符串,遍历字符串中的每一个字符。
如果当前字符是数字,则将其添加到数字中。如果当前字符是小数点,则标记为小数。如果当前字符是操作符,则将之前的数字压入数字栈中,并将当前操作符加入操作符栈中。
如果当前字符是左括号,则将其加入操作符栈中。如果当前字符是右括号,则将之前的数字压入数字栈中,并将操作符栈中的操作符进行计算,直到遇到第一个左括号。
最后,将数字栈中剩余的数字与操作符栈中的操作符进行计算,直到数字栈中只剩一个数字,即为最终结果。
其中,`calculate` 函数用于计算两个数字的四则运算及幂运算。
相关推荐
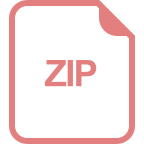
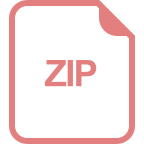














