vue3 + ant 实现下拉框选择器,该选择器可输入并进行模糊搜索
时间: 2024-04-10 16:33:11 浏览: 304
你可以使用 Vue 3 和 Ant Design Vue 来实现一个下拉框选择器,其中还可以输入并进行模糊搜索。这里是一个简单的示例代码:
首先,安装所需的依赖:
```
npm install vue@next ant-design-vue@next
```
然后,在你的 Vue 组件中,引入需要的组件和样式:
```vue
<template>
<a-select
v-model="selectedItem"
mode="tags"
placeholder="请输入关键字"
@search="handleSearch"
>
<a-select-option v-for="option in filteredOptions" :key="option">{{ option }}</a-select-option>
</a-select>
</template>
<script>
import { ref, reactive, watch } from 'vue';
import { Select } from 'ant-design-vue';
export default {
components: {
ASelect: Select,
ASelectOption: Select.Option,
},
setup() {
const options = ['Apple', 'Banana', 'Cherry', 'Durian', 'Elderberry'];
const selectedItem = ref([]);
const filteredOptions = reactive([]);
watch(selectedItem, (newVal) => {
console.log(newVal);
});
const handleSearch = (value) => {
filteredOptions.splice(0); // 清空数组
if (value) {
const searchResult = options.filter((option) =>
option.toLowerCase().includes(value.toLowerCase())
);
filteredOptions.push(...searchResult);
}
};
return {
selectedItem,
filteredOptions,
handleSearch,
};
},
};
</script>
<style>
@import '~ant-design-vue/dist/antd.css';
</style>
```
这个示例中,我们使用了 `a-select` 组件来实现下拉框选择器,并将 `mode` 属性设置为 "tags",以支持多选。`placeholder` 属性设置为 "请输入关键字",提供了一个搜索框用于输入关键字。
在 `@search` 事件中,我们通过过滤选项数组来实现模糊搜索功能,并将搜索结果更新到 `filteredOptions` 数组中。
最后,我们使用 `v-for` 指令来渲染 `a-select-option` 组件,将搜索结果展示为可选项。 `v-model` 指令用于绑定选中的项,可以通过 `selectedItem` 引用来获取或操作选中的项。
记得在组件中导入所需的组件和样式。这样,你就可以在你的 Vue 3 项目中使用这个下拉框选择器,并实现输入和模糊搜索了。
阅读全文
相关推荐





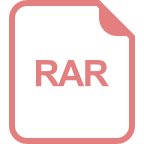











