用spring boot框架设计一款工时管理系统,生产工程与代码
时间: 2024-05-16 10:16:33 浏览: 12
好的,我来给您设计一款基于 Spring Boot 的工时管理系统。
首先,我们需要确定该系统的功能模块。一般来说,一款工时管理系统需要包含以下模块:
1.用户管理模块:包括用户登录、注册、密码重置等功能。
2.项目管理模块:包括项目的创建、修改、删除等功能。
3.任务管理模块:包括任务的创建、修改、删除等功能。
4.工时管理模块:包括工时的录入、查询、统计等功能。
5.报表管理模块:包括报表的生成、导出等功能。
6.权限管理模块:包括用户权限的管理等功能。
接下来,我们可以开始编写代码。首先,创建一个 Spring Boot 项目,并添加相关的依赖项,如 Spring MVC、Spring Data JPA、Thymeleaf 等。
在项目中创建实体类,如 User、Project、Task、TimeSheet 等。这些实体类用于映射数据库中的表,同时还需要编写对应的 Repository 接口,用于实现对数据库的增删改查操作。
然后,我们编写控制器类,用于处理用户请求。在控制器类中,我们可以定义一些请求处理方法,如处理用户登录、注册、项目创建、任务创建等请求。在这些方法中,我们可以调用对应的 Service 层方法,实现相应的业务逻辑。
在 Service 层中,我们可以定义一些服务方法,如创建用户、创建项目、创建任务等。在这些方法中,我们可以调用对应的 Repository 接口方法,实现对数据库的操作。
最后,我们可以编写视图模板,如登录页面、首页、项目管理页面、任务管理页面等,这些视图模板用于呈现给用户进行交互。
具体的代码实现过程可以参考以下示例代码:
1. 实体类
```
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
// getter 和 setter 方法
}
@Entity
@Table(name = "project")
public class Project {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Date startDate;
private Date endDate;
// getter 和 setter 方法
}
@Entity
@Table(name = "task")
public class Task {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String description;
// getter 和 setter 方法
}
@Entity
@Table(name = "timesheet")
public class TimeSheet {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private Long userId;
private Long projectId;
private Long taskId;
private Date date;
private Double hours;
// getter 和 setter 方法
}
```
2. Repository 接口
```
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
public interface ProjectRepository extends JpaRepository<Project, Long> {
}
public interface TaskRepository extends JpaRepository<Task, Long> {
}
public interface TimeSheetRepository extends JpaRepository<TimeSheet, Long> {
List<TimeSheet> findByUserIdAndDateBetween(Long userId, Date startDate, Date endDate);
}
```
3. Service 层
```
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public User findByUsername(String username) {
return userRepository.findByUsername(username);
}
public User createUser(User user) {
return userRepository.save(user);
}
}
@Service
public class ProjectService {
@Autowired
private ProjectRepository projectRepository;
public Project createProject(Project project) {
return projectRepository.save(project);
}
public List<Project> findAllProjects() {
return projectRepository.findAll();
}
}
@Service
public class TaskService {
@Autowired
private TaskRepository taskRepository;
public Task createTask(Task task) {
return taskRepository.save(task);
}
public List<Task> findAllTasks() {
return taskRepository.findAll();
}
}
@Service
public class TimeSheetService {
@Autowired
private TimeSheetRepository timeSheetRepository;
public List<TimeSheet> findByUserIdAndDateBetween(Long userId, Date startDate, Date endDate) {
return timeSheetRepository.findByUserIdAndDateBetween(userId, startDate, endDate);
}
public TimeSheet createTimeSheet(TimeSheet timeSheet) {
return timeSheetRepository.save(timeSheet);
}
}
```
4. 控制器类
```
@Controller
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/login")
public String login() {
return "login";
}
@PostMapping("/login")
public String doLogin(String username, String password, HttpSession session) {
User user = userService.findByUsername(username);
if (user != null && user.getPassword().equals(password)) {
session.setAttribute("user", user);
return "redirect:/";
} else {
return "login";
}
}
@GetMapping("/register")
public String register() {
return "register";
}
@PostMapping("/register")
public String doRegister(User user) {
userService.createUser(user);
return "redirect:/login";
}
@GetMapping("/logout")
public String logout(HttpSession session) {
session.invalidate();
return "redirect:/login";
}
}
@Controller
public class ProjectController {
@Autowired
private ProjectService projectService;
@GetMapping("/project")
public String project(Model model) {
List<Project> projects = projectService.findAllProjects();
model.addAttribute("projects", projects);
return "project";
}
@PostMapping("/project")
public String createProject(Project project) {
projectService.createProject(project);
return "redirect:/project";
}
}
@Controller
public class TaskController {
@Autowired
private TaskService taskService;
@GetMapping("/task")
public String task(Model model) {
List<Task> tasks = taskService.findAllTasks();
model.addAttribute("tasks", tasks);
return "task";
}
@PostMapping("/task")
public String createTask(Task task) {
taskService.createTask(task);
return "redirect:/task";
}
}
@Controller
public class TimeSheetController {
@Autowired
private TimeSheetService timeSheetService;
@GetMapping("/timesheet")
public String timeSheet(Model model, HttpSession session) {
User user = (User) session.getAttribute("user");
if (user != null) {
List<TimeSheet> timeSheets = timeSheetService.findByUserIdAndDateBetween(user.getId(), new Date(), new Date());
model.addAttribute("timeSheets", timeSheets);
}
return "timesheet";
}
@PostMapping("/timesheet")
public String createTimeSheet(TimeSheet timeSheet) {
timeSheetService.createTimeSheet(timeSheet);
return "redirect:/timesheet";
}
}
```
5. 视图模板
```
<!-- login.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<form action="/login" method="post">
<label>Username:</label>
<input type="text" name="username"><br>
<label>Password:</label>
<input type="password" name="password"><br>
<button type="submit">Login</button>
</form>
<a href="/register">Register</a>
</body>
</html>
<!-- register.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Register</title>
</head>
<body>
<h1>Register</h1>
<form action="/register" method="post">
<label>Username:</label>
<input type="text" name="username"><br>
<label>Password:</label>
<input type="password" name="password"><br>
<button type="submit">Register</button>
</form>
<a href="/login">Login</a>
</body>
</html>
<!-- project.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Project Management</title>
</head>
<body>
<h1>Project Management</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Start Date</th>
<th>End Date</th>
</tr>
</thead>
<tbody>
<tr th:each="project : ${projects}">
<td th:text="${project.id}"></td>
<td th:text="${project.name}"></td>
<td th:text="${project.startDate}"></td>
<td th:text="${project.endDate}"></td>
</tr>
</tbody>
</table>
<form action="/project" method="post">
<label>Name:</label>
<input type="text" name="name"><br>
<label>Start Date:</label>
<input type="date" name="startDate"><br>
<label>End Date:</label>
<input type="date" name="endDate"><br>
<button type="submit">Create Project</button>
</form>
</body>
</html>
<!-- task.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Task Management</title>
</head>
<body>
<h1>Task Management</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Description</th>
</tr>
</thead>
<tbody>
<tr th:each="task : ${tasks}">
<td th:text="${task.id}"></td>
<td th:text="${task.name}"></td>
<td th:text="${task.description}"></td>
</tr>
</tbody>
</table>
<form action="/task" method="post">
<label>Name:</label>
<input type="text" name="name"><br>
<label>Description:</label>
<input type="text" name="description"><br>
<button type="submit">Create Task</button>
</form>
</body>
</html>
<!-- timesheet.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Time Sheet</title>
</head>
<body>
<h1>Time Sheet</h1>
<table>
<thead>
<tr>
<th>User ID</th>
<th>Project ID</th>
<th>Task ID</th>
<th>Date</th>
<th>Hours</th>
</tr>
</thead>
<tbody>
<tr th:each="timeSheet : ${timeSheets}">
<td th:text="${timeSheet.userId}"></td>
<td th:text="${timeSheet.projectId}"></td>
<td th:text="${timeSheet.taskId}"></td>
<td th:text="${timeSheet.date}"></td>
<td th:text="${timeSheet.hours}"></td>
</tr>
</tbody>
</table>
<form action="/timesheet" method="post">
<label>Project ID:</label>
<input type="text" name="projectId"><br>
<label>Task ID:</label>
<input type="text" name="taskId"><br>
<label>Date:</label>
<input type="date" name="date"><br>
<label>Hours:</label>
<input type="text" name="hours"><br>
<button type="submit">Submit Time Sheet</button>
</form>
</body>
</html>
```
以上是一个简单的工时管理系统的设计和实现过程。当然,其中还有很多细节需要根据实际需求进行调整和完善。
相关推荐
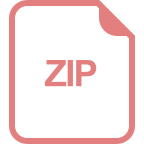
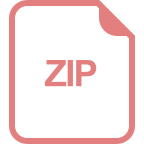
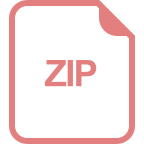















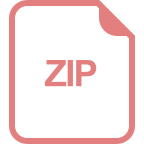