用c++绘制三角函数图像
时间: 2024-10-27 15:15:29 浏览: 27
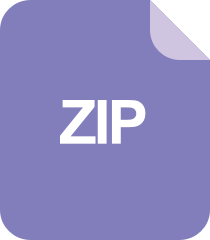
QT C++ 函数绘制器
在C++中,绘制三角函数图像通常涉及到图形库的使用,例如`SFML`、`Qt`或`OpenGL`等。这里我们选择一个轻量级的库`SFML`来演示如何创建一个基本的窗口并画出正弦波线。首先确保已经安装了SFML库。
```cpp
#include <SFML/Graphics.hpp>
const int windowWidth = 800;
const int windowHeight = 600;
void drawSineWave(sf::RenderWindow& window, float x, float amplitude, float frequency)
{
sf::Vertex vertices[windowWidth];
for (int i = 0; i < windowWidth; ++i)
{
float newX = static_cast<float>(i) * window.getSize().x / windowWidth;
float newY = amplitude * sin(2 * M_PI * frequency * newX);
vertices[i].position = sf::Vector2f(newX, windowHeight - newY); // 反转Y轴,因为我们在二维空间上是从下往上看
vertices[i].color = sf::Color::White;
}
window.draw(vertices, sf::Vocabulary::Lines, vertexCount);
}
int main()
{
sf::RenderWindow window(sf::VideoMode(windowWidth, windowHeight), "Sine Wave");
while (window.isOpen())
{
sf::Event event;
while (window.pollEvent(event))
{
if (event.type == sf::Event::Closed)
window.close();
}
// Clear the screen
window.clear();
// Draw the sine wave
drawSineWave(window, 0, 50, 0.01); // 设置振幅和频率
// Display the window contents
window.display();
}
return 0;
}
```
这段代码首先包含了SFML库,然后定义了一个窗口的尺寸。`drawSineWave`函数计算每个像素位置对应的正弦值,形成一条曲线。主循环里,我们不断处理窗口事件(如关闭请求),清除屏幕,然后调用`drawSineWave`函数并在窗口上显示出来。
要运行这段代码,你需要编译链接SFML库。对于初学者,推荐使用集成开发环境(IDE)如Code::Blocks或Visual Studio,它们通常提供了对第三方库的支持。
阅读全文
相关推荐
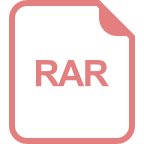
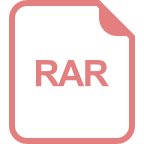















