使用python制作一个抽奖程序,点击开始开始滚动,点击停止停止滚动
时间: 2024-11-10 07:25:27 浏览: 27
制作一个简单的Python抽奖程序,你可以利用`random`模块来模拟抽奖过程,`threading`模块来实现并发的滚动效果。下面是一个基础的示例:
```python
import threading
import time
import random
class LotteryThread(threading.Thread):
def __init__(self, prizes, stop_event):
super().__init__()
self.prizes = prizes
self.stop_event = stop_event
self.is_running = False
def run(self):
self.is_running = True
while not self.stop_event.wait(1): # 每秒检查stop_event是否已触发
if self.is_running:
prize = random.choice(self.prizes)
print(f"恭喜,你抽到了:{prize}")
self.prizes.remove(prize)
def stop(self):
self.is_running = False
self.stop_event.set() # 触发stop_event,结束循环
def start_lottery(prizes):
stop_event = threading.Event()
lottery_thread = LotteryThread(prizes, stop_event)
lottery_thread.start()
def stop_lottery(lottery_thread):
lottery_thread.stop()
lottery_thread.join() # 等待线程结束
if __name__ == "__main__":
prizes = ["一等奖", "二等奖", "三等奖", "参与奖"]
start_lottery(prizes)
# 这里添加一个按钮,点击后调用stop_lottery停止抽奖
# 示例代码,实际应用中需要替换为按钮绑定的回调函数
while True:
user_input = input("请输入'开始'启动抽奖,输入'停止'停止抽奖:")
if user_input.lower() == '停止':
stop_lottery(lottery_thread)
break
elif user_input.lower() == '开始':
continue
else:
print("无效输入,请重新尝试.")
```
在这个例子中,我们首先定义了一个LotteryThread类,继承自`threading.Thread`。该类包含一个运行和停止方法,以及一个事件对象`stop_event`,用于在线程之间同步。
`start_lottery`函数启动抽奖线程,`stop_lottery`函数则是停止并等待线程结束。用户可以通过输入命令来启动或停止抽奖。
注意这只是一个基础版的抽奖程序,实际应用中可能还需要考虑UI设计、异常处理和交互体验等细节。
阅读全文
相关推荐









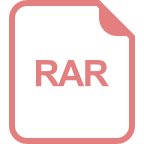







